All Computer Science Resources
Example Questions
Example Question #31 : Standard Data Structures
int a = 49;
int b = 6;
int c = 49 % 6;
What is the value of c
?
The modulus (or modulo) operator returns the remainder of a division statement. It only works with integer operands. Thus, it will only return an integer result. Six is able to divide forty-eight a maximum of eight times.
And then
Thus,
Example Question #32 : Standard Data Structures
int a = 5;
a += 3.14;
Using the language C++ or Java, what will the value of a
be after the above code snippet?
When using the plus-equals (+=) operator, the answer is cast to the type of the left-hand argument. Since a
was an int
, the value returned by the expression is converted to int
before it is stored in a
. Thus, the decimal point and any information behind the decimal is truncated.
Example Question #1 : Evaluating Numerical Expressions
Consider the following code:
int i = 85, j = 2, k = 4;
i--;
j++;
k -= 6;
i = i / j + i / k;
What is the value of i in the code above?
It is probably easiest to give a running commentary on the code in order to understand it. See the comments in bold below:
int i = 85, j = 2, k = 4;
i--;
// After this, i = 84
j++;
// After this, j = 3
k -= 6;
// After this, k = k - 6 or k = -2
/*
Consider this in several steps:
i / j = 84 / 3 = 28
i / k = 84 / -2 = -42
Thus, the final expression is:
i = 28 - 42 = -14
*/
i = i / j + i / k;
Example Question #32 : Primitive Data Types
Consider the code below:
int a = 24, b = 17, c = 5, d = 7;
b = b % d + a / b;
a %= b;
a -= 12;
What is the value of a at the end of the code above?
The easiest way to trace code like this is to provide running commentary in bold comments. See these below:
int a = 24, b = 17, c = 5, d = 7;
/*
The modulus (%) is the remainder of a division. So . . .
b % d = 17 % 7 = 3 (17 divided by 7 is 2 remainder 3.)
a / b = 1 (Remember, integer division truncates the decimal portion.)
Thus, we have:
b = 3 + 1 = 4
*/
b = b % d + a / b;
a %= b;
// This is the same as a = a % d, which is a = 24 % 4 = 0 (No remainder)
a -= 12;
// Remember that this is the same as a = a - 12, which is a = 0-12 or -12.
Example Question #2 : Evaluating Numerical Expressions
What is the Unicode value for the letter "W"?
U+007C
U+0057
U+0047
U+0087
U+005C
U+0057
The Unicode Standard is Java's attempt to simplify the translation of characters and symbols to input the computer can understand, while being able to work on all Java machines. The first 128 numbers (0-127, 0x0000-0x007F) are for basic Latin. Under this character encoding scheme, the letter 'W' is denoted by U+0057.
The standard for writing Unicode values is U+####, where the number is in hexadecimal format, not a base 10 number.
Example Question #3 : Evaluating Numerical Expressions
#include <iostream>
using namespace std;
int main()
{
bool bin=true;
int var=bin*2 +1;
int triple=5;
int final= (bin&var) &triple;
cout<<final;
return 0;
}
What is the output of this code?
false
2
1
0
3
1
Taking a look at the first line in main, the variable bin is defined as true, which we know to be 1. We see in the next line that the variable var is 2 * bin +1. The trick here is that you can actually do math with boolean values, so the value of var is 3.
The value of triple is 5.
The the variable final asks us to AND bin and var first, then AND triple.
To do this, we can convert all three numbers into binary notation to make it easier.
1 in binary is 001.
3 in binary is 011.
5 in binary is 101.
1&3 =1.
1&5=1.
Final answer is 1.
Example Question #35 : Primitive Data Types
int x = 2893;
- What does the expression " x / 10 " evaluate to?
- What does the expression " x % 10 " evaluate to?
- 289.3
- 893
- 289
- 3
- 3
- 289
- 289.3
- 3
- 289
- 2000
- 289
- 3
-
2893 / 10 = 289, since x is an int, it does not store the decimal value
- 2893 % 10 = 3 because the remainder of 2893/10 is 3
Example Question #36 : Primitive Data Types
int a;
a= 1+1 * 2 + 4 / 5;
System.out.println(a);
What would the code output?
3
2.4
0
3.8
2
3
The expression 1+1 * 2 + 4 / 5 can be rewritten as
1 + (1*2) + (4/5) = 1 + 2 + 0
4/5 = 0 because a is an int and when dividing ints, there are no decimals and since 4/5=0.8 we keep the ones digit which is zero.
Example Question #86 : Computer Science
Evaluate the mathematical equation?
You have to remember PEMDAS.
Multiplication and Division take precedence first
(double multiplied by an int equal a double)
Addition and Subtraction after
Example Question #1 : Evaluating String Expressions
string str = "Hello"
char newvar = str[4];
What is the value of newvar?
" "
'o'
'e'
'l'
'H'
'o'
The line, char newvar = str[4] is declaring a new variable which is of the type character. Further more, when it declares it, it is saying that it is the same as the character at the 4th location in the string above.
The value 4 actually represents the 5th value, since indices start at 0.
Therefore, at str[4] we have the character 'o'.
Certified Tutor
All Computer Science Resources
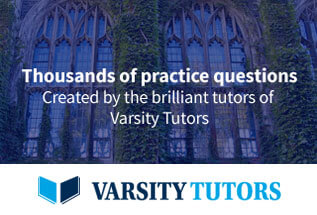