All Computer Science Resources
Example Questions
Example Question #1 : Evaluating String Expressions
String s = "abcdefghijklmnop";
String s2 = "";
for(int i = 0; i < s.length(); i += 2) {
s2 += s.charAt(i+1);
s2 += s.charAt(i);
}
What is the value of s2
at the end of the code above?
ponmlkjihgfedcba
aabbccddeeffgghhiijjkkllmmnnoopp
badcfehgjilknmpo
adfgmbnciephjkloa
adfgbnhciempjkloa
badcfehgjilknmpo
String s = "abcdefghijklmnop";
String s2 = "";
for(int i = 0; i < s.length(); i += 2) {
s2 += s.charAt(i+1);
s2 += s.charAt(i);
}
The key logic of this, of course, occurs in the loop. This loop can be thought of as looking at each pair of characters (hence the use of i += 2
as your increment condition). Thus, i
will be 0,2,4, . . . For 0, you will first place character 1 on s2
then character 0. Then, for 2, you will place 3 followed by 2. The pattern will continue. This is the same as saying that you will first do b
, then a
, next d
, then c
, and so forth. Therefore, for this code, you are flipping each pair of letters, thus giving you the answer badcfehgjilknmpo
.
Example Question #2 : Evaluating String Expressions
Consider the code below:
String s = "Logic!";
String s2 = "";
for(int i = 0; i < s.length(); i++) {
if(i != 0) {
s2 += " ";
}
s2 += s;
}
What is the value of s2
at the end of the code's execution?
Logic! Logic! Logic! Logic! Logic! Logic!
Logic! Logic! Logic! Logic! Logic! Logic!
Logic! Logic! Logic!
L L L L L L o o o o o o g g g g g g i i i i i i c c c c c c ! ! ! ! ! !
LoGiC! LoGiC! LoGiC! LoGiC! LoGiC! LoGiC!
Logic! Logic! Logic! Logic! Logic! Logic!
for(int i = 0; i < s.length(); i++) {
if(i != 0) {
s2 += " ";
}
s2 += s;
}
Let us focus on the main for loop that is used in this code. Notice that the loop will run from 0 to the length of the string s
. This string is 6 long. Therefore, the loop will run 6 times. The if
statement adds a space to the string on every iteration except for the first. Therefore, the code copies the string 6 times, each copy being separated by a space.
Example Question #2 : Evaluating String Expressions
Consider the following code:
What is the output of the method call mystery("Green eggs and ham")?
Green,eggs,and,ham,
Green eggs and ham
G,r,e,e,n,e,g,g,s,a,n,d,h,a,m,
Greeneggsandham,
ham,
Green,eggs,and,ham,
The method String.split() splits a String into an array of Strings separated according to the expression within the method argument. The expression String.split("\\s+") splits the String at every whitespace character. The "for" loop concatenates the elements of the String array together, separated by a comma.
Example Question #3 : Evaluating String Expressions
What is the output of the following code?
System.out.println( (1+2) + (3-1) + new Integer(5));
Syntax error, will not run
36
325
10
45
10
When evaluating a string expression with integers, arithmatic addition is performed if the integers are not separated by string Objects. Since all of the integers and operations in this expression are not separated by String objects, arithmatic operations are performed on the integers and the final airthmatic result is printed to the console.
Example Question #4 : Evaluating String Expressions
What is the output of the following code?
System.out.println((2*2) + " foo " + (3-1) + new Integer(5) + "3-2");
4 foo 253-2
4 foo 251
4 foo 8
4 foo 73-2
12 foo
4 foo 253-2
The presence of the "foo" String in the middle of the expression makes it such that arithmatic operations are performed separately on the left and right sides of the String. It causes each plus sign to perform concatenation instead of addition between terms.
Example Question #1 : Evaluating String Expressions
What is the best way to print out a String variable into a sentence in Swift (iOS)?
var str = "Hello"
println("This string says \(str)")
var str = "Hello"
println("This string says " + str)
var str = "Hello"
println("This string says str")
var str = "Hello"
println("This string says Hello")
var str = "Hello"
println("This string says \(str)")
The \(str) is the best way in Swift. This notation allows for a value of a variable to be input into the sentence. The "+" notation is also correct but is not the best way for Swift. The other two answers print out the words "str" and "Hello" instead of the value of the variable.
Example Question #4 : Evaluating String Expressions
Which of the following will cause the Java compiler to throw an error?
String s = String("Hello");
String s = "Hello" + 5;
String s = new String("Hello");
String s = "Hello";
String s = null;
String s = String("Hello");
The answer is E. E will throw cause Java to throw an error.
The question tests the students' syntactical knoweldge of Java, as well as the meaning of Java throwing a compiler error. Before running a piece of Java code, it must be compiled. The compiler converts the code into a language the machine understands, but more importantly for this example it catches any syntactical errors that the user might have made.
Answer A is correct, as you need the new keyword to initialize a String as an Object, so the line of code will throw an error.
Answer B is incorrect, as Strings can also be initialized as a primitive type.
Answer C is incorrect, as it is possible to give s a null reference.
Answer D is incorrect, as Java automatically casts the integer 5 to a string, effectivelly performing the following operation: "Hello" + "5". This is called implicit casting. This is a feature of the Java language, but for example, not of Python.
Answer E is incorrect, as Strings are also Objects in Java and can be initialized with the new keyword.
Example Question #5 : Evaluating String Expressions
Suppose you have the following lines of code:
Object integerObj = new Integer(2);
System.out.println((String) integerObj);
What will be the output resulting from running the lines of code?
No output. An exception is thrown of type ClassCastException
two
"2"
A compiler error will be thrown
2
No output. An exception is thrown of type ClassCastException
You cannot cast an Object of type Integer to String. The tricky part here is that the compiler will not be able to catch this because it is integerObject has type Object. Had it been of type Integer, a compiler error would be thrown. Thus, the code fragment is allowed to run but an exception is thrown at runtime instead.
Additionally, the option "two" does not make any sense, because even if 2 was cast to a String, the output would be "2", and not "two."
Also, the option ""2"" does not make sense because the quotes are not displayed.
Example Question #94 : Computer Science
int currentYear = 2016;
bool leapYear;
if (currentYear % 4 == 0) {
leapYear = true;
} else {
leapYear = false;
}
febDays = leapYear ? 28 : 29
Based on the code above, what value will febDays
have?
The ternary operator works as follows:
(condition to be checked) ? (value to return if true) : (value to return if false)
The answer is purposely logically incorrect to force you to consider the structure of the code. While 2016 is obviously a leap year where February should have 29 days, the ternary operator used is set to return 28 days if true and 29 if false. So in this case, febDays
will be 28.
Example Question #1 : Evaluating Boolean Expressions
Consider the following code:
int[] a = {8,4,1,5,1,5,6,2,4};
int b = 1;
boolean[] vals = new boolean[a.length];
for(int i = 0; i < a.length; i++) {
vals[i] = (a[i] - 1 > 4);
}
for(int i = 0; i < vals.length; i++) {
String s = "Duns Scotus";
if(!vals[i]) {
s = "Mithrandir";
}
System.out.println(s);
}
What is the output for the code above?
None of the others
Duns Scotus
Mithrandir
Mithrandir
Mithrandir
Mithrandir
Mithrandir
Duns Scotus
Mithrandir
Mithrandir
Duns Scotus
Mithrandir
Mithrandir
Duns Scotus
Mithrandir
Duns Scotus
Mithrandir
Duns Scotus
Duns Scotus
Duns Scotus
Duns Scotus
Duns Scotus
Duns Scotus
Duns Scotus
Duns Scotus
Duns Scotus
Duns Scotus
Mithrandir
Duns Scotus
Duns Scotus
Duns Scotus
Duns Scotus
Duns Scotus
Mithrandir
Duns Scotus
Duns Scotus
Duns Scotus
Mithrandir
Mithrandir
Mithrandir
Mithrandir
Mithrandir
Duns Scotus
Mithrandir
Mithrandir
Begin by getting a general idea of the loop logic being executed. For each execution of the first loop, you are assigning a boolean to the vals array. Now, you could rewrite the code a little to make the math easier:
a[i] - 1 > 4
really is the same as:
a[i] > 5
Now, the only values for which this is true are:
8 and 6 (the first and the 7th values in a).
Now, looking at the second loop, the value s will be "Duns Scotus" for every value that is true in vals. This is because the if statement checks for !vals[i]. Thus, the first and the seventh values alone will be "Duns Scotus". Everything else will be "Mithrandir".
Certified Tutor
All Computer Science Resources
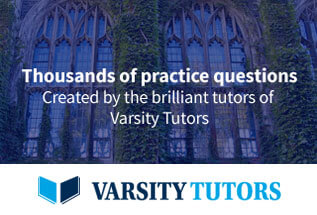