All Computer Science Resources
Example Questions
Example Question #91 : Standard Data Structures
Which of the following is NOT a difference between the Array class and the ArrayList class in Java?
Arrays have a fixed size at creation, while ArrayLists can grow as needed.
To find the number of spots in an Array, you append .length
to the end of it. To find the number of spots in an ArrayList, you use the size()
method.
You have to manually add things to an Array by calling a command similar to my_array[some_number] = some_value
. With ArrayLists, you use the add()
method.
All of the other answer choices are differences between Arrays and ArrayLists in Java.
Arrays can store both primitives and Objects, while ArrayLists can only store Objects.
All of the other answer choices are differences between Arrays and ArrayLists in Java.
In Java, Array is a fixed length data structure, while ArrayList is a variable length Collection Class (other Collection class members include HashMap and HashSet). This means that an Array cannot change its size once it's made; a whole new Array must be made. ArrayLists can change size at will.
Arrays can reference both primitives (like int
) and types (like Integer
). ArrayLists can only reference types, not primitives. Through a method called "Autoboxing", it can appear that an ArrayList stores a primitive, but what it really is doing is automatically masking the primitive with the type it's like so that it works with the ArrayList.
During the creation of an Array, the .length
value is assigned, so whenever you append .length
to the end of an array, you get the length (which will never change for the given Array). For ArrayLists, you use the .size()
function, which returns the length of the ArrayList. Because the ArrayList size is variable, it is a function (which is why the parenthesis are there at the end).
Java provides the add() function for ArrayLists, which, as the name implies, adds the argument to the ArrayList. Arrays, on the other hand, do not have functions to add elements. To add something to an Array, you'd call something similar to this:
int[] my_array = new int[10];
my_array[0] = 5;
Example Question #91 : Standard Data Structures
Write a program that iterates through this data structure and prints the data (choose the best answer):
List<List<String>> listOflistOfStrings = new ArrayList<ArrayList<String>>();
for (int i = 0; i < listOflistOfStrings.size(); i++) {
List<String> listOfStrings = listOflistOfStrings.get(i);
for (int j = 0; j < listOfStrings.size(); j++) {
System.out.println(listOfStrings.get(j));
}
}
for (int i = 0; i < listOflistOfStrings.size(); i++) {
List<String> listOfStrings = listOflistOfStrings.get(i);
for (int j = 0; j < listOflistOfStrings.size(); j++) {
System.out.println(listOfStrings.get(j));
}
}
for (List<String> l : listOflistOfStrings) {
List<String> listOfStrings = l;
for (List<String> s : l) {
System.out.println(s);
}
}
for (List<String> l : listOflistOfStrings) {
List<String> listOfStrings = l;
for (String s : l) {
System.out.println(s);
}
}
for (List<String> l : listOflistOfStrings) {
List<String> listOfStrings = l;
for (String s : l) {
System.out.println(s);
}
}
The correct answer uses a ForEach loop. A ForEach loop is recommended for iterating through Lists because Lists contain iterators. ForEach loops use the iterator to iterate through the List. One of the answers used a regular For loop, while the answer was correct, it was not the best choice.
Example Question #92 : Standard Data Structures
int foo[] = {1, 2, 3, 4, 5};
number = 100 + foo[4];
What is the value of number
?
Error.
The answer is 105 because arrays are zero indexed. This means that the first position has the subscript 0, the second subscript 1, and so on. 5 is in the fifth space, located at subscript 4. We would access it by saying foo[4]. As another example, if we wanted to access one, we would say foo[0].
Example Question #93 : Standard Data Structures
Consider the following code:
int[] vals = {6,1,41,5,1};
int[][] newVals = new int[vals.length][];
for(int i = 0; i < vals.length; i++) {
newVals[i] = new int[vals[i]];
for(int j = 0; j < vals[i];j++) {
newVals[i][j] = vals[i] * (j+1);
}
}
What does the code above do?
It sums the values in vals, placing the outcome in newVals.
The code creates a 2D array that contains all multiples from 1 to 5 for the members of vals.
It creates a 2D matrix with the vals array for every row.
The code creates a 2D array that contains the multiples for the members of vals, using each of those values to determine the number of multiples to be computed.
It performs a matrix multiplication on vals and newVals, storing the final result in newVals.
The code creates a 2D array that contains the multiples for the members of vals, using each of those values to determine the number of multiples to be computed.
Let's look at the main loop in this program:
for(int i = 0; i < vals.length; i++) {
newVals[i] = new int[vals[i]];
for(int j = 0; j < vals[i];j++) {
newVals[i][j] = vals[i] * (j+1);
}
}
The first loop clearly runs through the vals array for the number of items in that array. After this, it creates in the newVals 2D array a second dimension that is as long as the value in the input array vals. Thus, for 41, it creates a 3rd row in newVals that is 41 columns wide.
Then, we go to the second loop. This one iterates from 0 to the value stored in the current location in vals. It places in the given 2D array location the multiple of the value. Think of j + 1. This will go from 1 to 41 for the case of 41 (and likewise for all the values). Thus, you create a 2D array with all the multiples of the initial vals array.
Example Question #94 : Standard Data Structures
Consider the following code:
public static void main(String[] args) {
int[] vec = {8,-2,4,5,-8};
foo(vec);
}
private static void foo(int[] x) {
for(int i = 0; i < x.length; i++) {
int y = Math.abs(x[i]);
for(int j = 0; j < y; j++) {
System.out.print(x[i] + " ");
}
System.out.println();
}
}
Which of the following represents a possible output for the program above?
8 8 8 8 8 8 8 8
4 4 4 4
5 5 5 5 5
64
-4
16
25
-64
64
4
16
25
64
8 8 8 8 8 8 8 8
-2 -2
4 4 4 4
5 5 5 5 5
-8 -8 -8 -8 -8 -8 -8 -8
37
8 8 8 8 8 8 8 8
-2 -2
4 4 4 4
5 5 5 5 5
-8 -8 -8 -8 -8 -8 -8 -8
In this code's loop, notice that it takes the absolute value of each element. This is done on the line:
int y = Math.abs(x[i]);
This value is then used for the second loop, which goes for y times, each time outputting the value of the given member of the original array—but now with its particular sign value. Thus, even numbers like will be output multiple times (i.e. 8). This is done line by line for each member of the parameter array.
Example Question #1 : Arrays
Consider the following code:
public static void main(String[] args) {
double[][] matrix = {{1,6,7},{1,4,5}};
graphics(matrix);
}
private static double graphics(double[][] x) {
double r = 0;
for(int i = 0; i < x.length; i++) {
for(int j = 0; j < x[i].length; j++) {
r += x[i][j] * (i + 1);
}
}
return r;
}
What is the return value for graphics in the code below:
double[][] matrix = {{1,6,7},{1,4,5}};
graphics(matrix);
The graphics method takes the 2D array matrix and then runs through each element. This is the point of the double for loop in the method itself. Notice that for each element it does several things. First of all, it is clearly accumulating a value into the variable r. Now, for each element, you are adding:
x[i][j] (the current value in the 2D array iteration)
TIMES
(i + 1), or, the current row number. Thus, for the data given you are doing the following:
1* 1 + 1 * 6 + 1 * 7 + 2 * 1 + 2 * 4 + 2 * 5 = 34
Example Question #95 : Standard Data Structures
For the following question, consider the following code:
public static void main(String[] args)
{
double[] vec = {4,8,10,18};
System.out.println(fun(vec));
}
privatestaticdouble fun(double[] x)
{
double p = 0;
for(int i = x.length-1; i > -1; i--)
{
p += x[i];
}
return p / x.length;
}
Which of the following is a possible output for this program?
First of all, you can eliminate the following two options very quickly, as the fun method returns a double value:
[D@4b71bbc9]
[4,9,11,19]
These are trying to trick you into thinking that it returns an array. (The first value is really what would be the output, since there is no toString defined for the double array by default.)
Now, in fun, the loop simply runs through the values in the array, summing those up. Notice, it runs "backwards" through the array, from the end to the beginning. It then divides by the length of the array, giving you the average of the values in the array.
Example Question #2 : Arrays
Consider the following code:
String s = "I read logic for fun!";
boolean[] b = {true,false,true,false,false,true,true,false,true,false,true,false,false,true,false,true,true,false,true,false,false};
for(int i = 0; i < s.length(); i++) {
char c = s.charAt(i);
if(!b[i]) {
c = Character.toUpperCase(c);
} else {
c = Character.toLowerCase(c);
}
System.out.print(c);
}
What is the output for this function code?
i rEAd LoGiC fOr FuN!
I ReAd lOgIc fOr FuN!
I read LOGIC for FUN!
The code will throw an exception.
I ReAd LoGiC FoR FuN!
i rEAd LoGiC fOr FuN!
This code is using what are called parallel arrays. The boolean array has one value for each character in the String s. Notice the logic in the loop. There is a condition:
if(!b[i]) {
c = Character.toUpperCase(c);
} else {
c = Character.toLowerCase(c);
}
This means that if the boolean is false, then you will make the given letter upper case. (This is because of the ! in the condition. Be careful!) Otherwise, you make it lower case.
Carefully walking through the code, you will get:
i rEAd LoGiC fOr FuN!
Example Question #92 : Standard Data Structures
Given the following in C++:
int * data = new int[12];
Pick an expression that is equivalent to : data[5];
(data+5)*
&(data+5)
data + 5
*(data +5);
(data + 5)&
*(data +5);
Let's look at this line of code:
int * data = new int[12]
An int pointer is created and an array of size 12 is assigned to it.
When data[5] is called, the "data" is dereferenced and the value of the 6th position is returned.
The only one of the choices that does this is
*(data + 5)
In line above, the pointer is incremented ot the 6th position and is then dereferenced to get the value.
If the code was
*data
The first item at the first position will be returned.
*(data+1)
This will return the item and the second position and so on.
Example Question #91 : Standard Data Structures
TWO DIMENSIONAL ARRAYS
Given the following initialized array:
int fourth;
int[][] myArray = { {1, 2, 3},
{4, 5, 6},
{7, 8, 9} };
Using myArray, how can I store in variable "fourth", the number 4?
fourth = myArray[2][1];
fourth = myArray[1][1];
fourth = myArray[1][0];
fourth = myArray[0][1];
fourth = myArray[1][0];
When a two dimensional array is created and initialized, the way to access the items inside the matrix is by calling the array with the row and column (i.e. myArray[ROW][COLUMN]). Keeping in mind that arrays start at 0, the number four would be in row 1, column 0. Therefore to save that number into the variable "fourth" we'll do the following:
fourth = myArray[1][0];
*Note: myArray[1][0] is not the same as myArray[0][1].
myArray[1][0] =4 because it is the item located at row=1 and column = 0.
myArray[0][1] =12 because it is the item located at row=0 and column = 1.
All Computer Science Resources
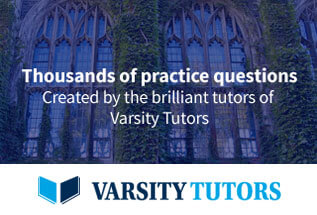