All Computer Science Resources
Example Questions
Example Question #2 : Evaluating Boolean Expressions
Consider the following code:
int a = 14;
int b = -15;
int c = 22;
int d = 11;
if(a > 12 && b < -14 && c < d) {
System.out.println("YAY!!!!");
} else if (d - 20 < b) {
System.out.println("GO DO MORE PROGRAMMING!");
} else if(a + 12 >= c) {
System.out.println("This is vexing......");
} else {
System.out.println("This is very fun!");
}}
What is the output for the code above?
This is very fun!
YAY!!!!
No output appears.
GO DO MORE PROGRAMMING!
This is vexing......
This is vexing......
Consider each condition:
The first if statement has: a > 12 && b < -14 && c < d
While it is true that 14 > 12 and -15 < -14, it is not true that 11 < 22. Therefore, the conjunction of all of these cannot be true. For &&, all of the expressions must be true.
Next, consider: d - 20 < b
This is really: 11 - 20 < -15 or -9 < -15. This is not true either.
Next, consider: a + 12 >= c
This is really: 12 + 12 >= 22 or 24 >= 22. This is true. Therefore, your output is "This is vexing......"!
Example Question #3 : Evaluating Boolean Expressions
Consider the code below:
for(int i = 0; i < 10; i++) {
if(i % 2 == 0) {
System.out.println("This is my favorite case!");
} else if(i + 6 > 12 && i % 3 == 1) {
System.out.println("There once was a pool in the back yard!");
} else {
System.out.println("Well, at least it is something...");
}
}
What is the output for the code above?
This is my favorite case!
Well, at least it is something...
This is my favorite case!
Well, at least it is something...
This is my favorite case!
There once was a pool in the back yard!
Well, at least it is something...
This is my favorite case!
This is my favorite case!
Well, at least it is something...
This is my favorite case!
Well, at least it is something...
This is my favorite case!
Well, at least it is something...
This is my favorite case!
Well, at least it is something...
This is my favorite case!
This is my favorite case!
There once was a pool in the back yard!
This is my favorite case!
This is my favorite case!
Well, at least it is something...
This is my favorite case!
Well, at least it is something...
This is my favorite case!
Well, at least it is something...
This is my favorite case!
Well, at least it is something...
This is my favorite case!
Well, at least it is something...
This is my favorite case!
Well, at least it is something...
This is my favorite case!
Well, at least it is something...
This is my favorite case!
Well, at least it is something...
This is my favorite case!
There once was a pool in the back yard!
This is my favorite case!
Well, at least it is something...
This is my favorite case!
Well, at least it is something...
This is my favorite case!
Well, at least it is something...
This is my favorite case!
Well, at least it is something...
This is my favorite case!
This is my favorite case!
There once was a pool in the back yard!
Well, at least it is something...
This is my favorite case!
Well, at least it is something...
This is my favorite case!
Well, at least it is something...
This is my favorite case!
Well, at least it is something...
This is my favorite case!
There once was a pool in the back yard!
This is my favorite case!
Well, at least it is something...
First of all, note that your loop will run for values from 0 to 9, inclusive.
Now, the first condition is relatively easy:
i % 2 == 0
This is true when the number i
is even. When it is divisible by 2, the remainder will be 0. (Recall that the modulus gives you the remainder.)
Now, the next two else
conditions will execute only for odd numbers. The first one can be rewritten:
i > 6 && i % 3 == 1
This means that we need all of the numbers from 7 to 9 that have a remainder of 1 when divided by 3. This only applies to i = 7
. This will be the eighth line output. Therefore, you know that you will have alternating lines saying, "This is my favorite case!" All of the other ones will be "Well, at least it is something...", except for the eighth line, which will be "There once was a pool in the back yard!"
Example Question #2 : Evaluating Boolean Expressions
Consider the code below:
int i = 45, j = -12;
String s = "This is great!";
String s2 = "Where are you?";
if(s2.charAt(4) == ' ' && (i < 4 || j > -43) && !s2.equals("WOW!!!")) {
System.out.println("Happy days are here again!");
} else if (s2.charAt(5) == 's') {
System.out.println("Well, we are here at least.");
} else if (j + 12 != 0) {
System.out.println("The stock market is up today!");
} else if(i - 20 < 100) {
System.out.println("Where will we be having lunch tomorrow?");
} else {
System.out.println("But here you go!");
}
What is the output for the code above?
But here you go!
Happy days are here again!
Where will the group be having lunch tomorrow?
The stock market is up today!
Well, we are here at least.
Where will the group be having lunch tomorrow?
The easiest way to begin is by looking at the general form of the first if
statement. Notice that it is made up of three expressions that have a boolean AND
between them. This means that if any of those members is false, the whole thing will be false. Well, consider the very first term:
s2.charAt(4) == ' '
This is asking about the fifth character in the string s2
. (Remember, the index starts at 0.) Well, this is true for s
but not for s2
, which has 'e
' for its fifth member. Thus, we know that the first if
must be false.
Now, to the second:
s2.charAt(5) == 's'
This also is not true, for the sixth character in s2
is ' '
.
The third if
is also false. j + 12
is equal to 0!
However, it is indeed the case that (45 - 20 < 100)
is true. Therefore, the fourth if
statement will execute, giving you the output:
Where will the group be having lunch tomorrow?
Example Question #2 : Evaluating Boolean Expressions
Consider the code below:
int i = 12, j = -5, k = 103;
if(i < 15 && j >= -4) {
System.out.println("You should take more philosophy classes!");
} else if(!(i < 100 && k > 4)) {
System.out.println("I love medieval studies!");
} else if(!(i == 3 && k == 4)) {
System.out.println("My favorite philosopher is Radulphus Brito, of course!");
} else if(i != 4 && k == 102) {
System.out.println("My favorite philosopher is Johann Fichte.");
} else {
System.out.println("I am going to cut the grass tomorrow.");
}
What is the output for the code above?
I love medieval studies!
My favorite philosopher is Johann Fichte.
My favorite philosopher is Radulphus Brito, of course!
I am going to cut the grass tomorrow.
You should take more philosophy classes!
My favorite philosopher is Radulphus Brito, of course!
Let us consider each conditional in turn. When we reach one that is true, we know our output.
First Conditional: i < 15 && j >= -4
Recall that you must have both of these true in order for the whole expression to be true. Well, while it is the case that , it is not true that
. Thus, this conditional is false.
Second Conditional: !(i < 100 && k > 4)
For this, you must first attempt the expression in parentheses. Both of these are true. However, !(true)
is false. (It makes sense in English: "That is not true!" This means, "That is false!")
Third Conditional: !(i == 3 && k == 4)
Well, here, we know that both 12 == 3
and 103 == 4
are both false. Therefore, the expression is !(false)
. Therefore, it is true! This is your output.
Example Question #1 : Evaluating Boolean Expressions
Consider the code below:
char c = 'M';
String s = "James of Metz";
String s2 = "Albert of Sazony";
int i = 14;
if(i > 34 || c < 'B') {
System.out.println("I love Duns Scotus!");
} else if(s.charAt(9) == c) {
System.out.println("I read William of Ockham yesterday!");
} else if(!(i < 100 || s.equals("James of Metz"))) {
System.out.println("Well, I call William of Ockham 'Bill' because we're BFFs.");
} else if(s2.equalsIgnoreCase("Al")) {
System.out.println("I was reading Simon of Faversham, and he is very logical.");
} else {
System.out.println("There is nobody like Peter of Spain for a good logic read.");
}
What is the output for the code above?
I read William of Ockham yesterday!
I was reading Simon of Faversham, and he is very logical.
I love Duns Scotus!
There is nobody like Peter of Spain for a good logic read.
Well, I call William of Ockham 'Bill' because we're BFFs.
I read William of Ockham yesterday!
Let's consider the cases in order until we find our matching true expression.
Conditional 1: i > 34 || c < 'B'
All we need is for one of these to be true for the whole expression to be true (given the nature of the logical OR represented by ||
. However, neither of these are true.
Conditional 2: s.charAt(9) == c
If you carefully count the letters in the String s
, you will see that index 9 (i.e. the tenth character) is M
. Therefore, this is equal to c
. Thus, you know that your program will output I read William of Ockham yesterday!
Example Question #2 : Evaluating Boolean Expressions
What does the expression (7 != 14) evaluate to?
14
7
True
2
False
True
The "!" symbol means not. Therefore, 7 does not equal 14 is true.
Example Question #3 : Evaluating Boolean Expressions
boolean x;
int y;
x = false;
y = 10;
x = !(y < 10 || y > 10);
System.out.println ( x );
What is printed?
True
False
10
5
x
True
The expression !(y < 10 || y > 10) evaluates to (y >= 10 && y <= 10).
Since y = 10, the expression evaluates to true.
Example Question #1 : Evaluating Boolean Expressions
What does this statement return?
int a = 2
int b = 3
a == b
false
true
indeterminate
null
false
a and b are both primitive types of integer. a is equal to 2 and b is equal to 3. 2 does not equal 3, so the answer is false.
Example Question #101 : Computer Science
Which is correct?
String a = "2"
String b = "2"
Is a == b correct? Or is a.equals(b) correct?
Neither is correct
a == b
Both answers have the same result
a.equals(b)
a.equals(b)
Since both objects are not primitive types, double equals, ==, would return whether the pointers of the type objects are equivalent. The string object requires the string equals method which is String.eq("string")
Example Question #11 : Evaluating Boolean Expressions
Which is equivalent?
int a = 5
int b = 10
int c = 25
int d = 15
- (a + b) == c
- (a * b) == d
- (a + b) == d
- (a * c) == b
4
2
3
1
3
3 is the correct answer because a = 5 and b = 10 so 5 + 10 = 15 which is d.
Certified Tutor
All Computer Science Resources
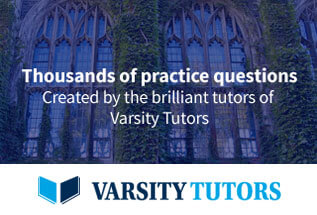