All Computer Science Resources
Example Questions
Example Question #1 : Debugging
Consider the following code:
public static class Rectangle {
private double width, height;
public Rectangle(double w,double h) {
width = w;
height = h;
}
public double getArea() {
return width * height;
}
public double getPerimeter() {
return 2 * width + 2 * height;
}
}
public static class Square extends Rectangle {
public Square(double s) {
super(s,s);
}
}
public static void main(String[] args) {
Rectangle[] rects = new Rectangle[6];
for(int i = 0; i < 6; i++) {
if(i % 2 == 0) {
rects[i] = new Rectangle(i+10,i + 20);
} else {
rects[i] = new Square(i+20);
}
}
Square s = rects[1];
}
What is the error in the code above?
There is an error in the declaration of the Rectangle
array.
There is an array overrun.
You need to use "implements", not "extends" for the class Square
.
You cannot assign a Square
object to an array of Rectangle
objects.
The final assignment operation cannot be done.
The final assignment operation cannot be done.
This code fills up the 6 member array with alternating Rectangle and Square objects. You can do this because the Square class is a subclass of Rectangle. That is, since Squares are Rectangles, you can store Square objects in Rectangle variables. However, even though rects[1]
is a square, you CANNOT immediately reassign that to a Square object. The code has now come to consider all of the objects in the array as being Rectangle objects. You would need to explicitly type cast this to get the line to work:
Square s = (Square)(rects[1]);
Example Question #1 : Compile Time Errors
What is the error in the following code?
int val1 = -14,val2 = 4;
final int val3 = 9;
double val4 = 4.1;
double val5 = 3.1;
val1 = val2 * val3;
val3 = val1 * 12;
val5 = val1 - val3;
val4 = val2 + val5;
You cannot assign the new integer value to val3
.
You cannot multiply val3
by val2
.
You cannot perform mixed addition or subtraction with doubles and integers.
You must define val2
on its own line.
You cannot assign the result of an integer subtraction to val5
.
You cannot assign the new integer value to val3
.
The only error among the options given is the fact that this code assigns a new value to the variable val3
, which is defined as a constant. (This is indicated by the keyword final
before the rest of its declaration.) You cannot alter constants once they have been declared. Thus, the following line will cause a compile-time error:
val3 = val1 * 12;
Example Question #2 : Debugging
public static void main(String[] args) {
int[] x = {3,4,4,5,17,4,3,1};
int[] y = remove(x,4);
for(int i = 0; i < y.length; i++) {
System.out.print(y[i] + " ");
}
}
public static boolean remove(int[] arr, int val) {
boolean found = false;
int i;
for(i = 0; i < arr.length && !found; i++) {
if(arr[i] == val) {
found = true;
}
}
if(found) {
for(int j = i; j < arr.length;j++) {
arr[j - 1] = arr[j];
}
arr[arr.length - 1] = 0;
}
return found;
}
What is the error in the code above?
There are multiple options to delete, which is not supported by the function
There is a type mismatch in one of the assignments
The output loop in main
will overrun the array y
In the remove
method, there is a variable that has not been initialized before being used
There is no error in the code
There is a type mismatch in one of the assignments
The problematic line is this one:
int[] y = remove(x,4);
Notice that the variable y is defined as an array. Now, it is tempting to think (without looking too closely) that the remove
method returns the array after the removal has been accomplished; however, this is not how the logic works in the remove
method. Instead, it returns a boolean indicating whether or not this removal was successful or not (i.e. it tells you whether or not it actually found the value). Therefore, you cannot make an assignment like the one above, for the two types are not the same. That is, y
is an integer array, while remove
returns a boolean value!
Example Question #4 : Debugging
public interface ServerInstance {
byte[] readBytes();
boolean writeBytes(byte[]b);
boolean wake();
boolean status();
void sleep();
}
public class MyHost implements ServerInstance {
boolean running = false;
public boolean wake() {
// Other logic code here...
return running;
}
public boolean status() {
// Other logic code here...
return running;
}
public byte[] readBytes() {
byte[] buffer = null;
// Other logic code here...
return buffer;
}
public void sleep() {
// Other logic code here...
running = false;
}
public byte[] writeBytes(byte[] b) {
// Other logic code here...
return b;
}
// Other methods...
}
What is the error in the code above?
The writeBytes
method is not properly defined
The word extends
should be used, not implements
The readBytes
method initializes the variable buffer
to null
There is no error
The sleep
method does not return anything
The writeBytes
method is not properly defined
When you implement an interface, all of the methods defined in that interface must be written in the class that is proposing to be such an implementation. (Or, if they are not implemented there, you need to involve abstract classes—but that is not our concern here.) The methods must match the prototypes proposed in the interface. In the example code, ServerInstance
has a method writeBytes
that returns a boolean value. However, the MyHost
class has implemented this method as returning a byte[]
value. Since you cannot have different types of return values for methods with the same parameter set, Java interprets this as being the proposed implementation for writeBytes(byte[] b)
, and this method must return a boolean if MyHost
is to implement ServerInstance
.
Example Question #5 : Debugging
public static void foo() {
int x = 10; y = 21, z = 30;
int[] arr = null;
for(int i = 0; i < y; i+= 4) {
arr = new int[i / 5];
}
for(int i = 0; i < x; i++) {
arr[i] = z / i;
}
for(int i = 0; i < z; i++) {
arr[i] = z * i;
}
}
Which of the following lines of code will cause a compile-time
error?
arr[i] = z / i;
arr = new int[i / 5];
arr[i] = z * i;
None of these lines will cause a compile-time
error
int x = 10; y = 21, z = 30;
int x = 10; y = 21, z = 30;
An error in compilation occurs before any code even attempts to execute. Thus, it is primarily a syntactical error in the code. In the selection above, the line
int x = 10; y = 21, z = 30;
has a semicolon right after 10.
This causes an error in the code directly following on this, for the code
y = 21, z = 30;
is not valid Java code.
There are other errors in this code. arr[i] = z / i;
will cause a divide by 0 error when i is 0. Also, arr[i] = z * i;
will overrun the bounds of the array arr
. However, these are not compile-time errors—i.e. errors that occur before the code is even able to run!
Example Question #1 : Debugging
class Base{};
class Derived : public Base{
public:
void method(){ cout<< "method1\n"; }
};
class Derived2 : public Base{
public:
void method() { cout<< "method2\n"; }
};
int main(){
Base* bp = new Derived();
Derived2* d2p = bp;
d2p -> method();
}
What is the result of compiling and running the program in C++?
The program compiles and runs to completion without printing anything
The program compiles and crashes when it runs.
The program compiles and runs, printing "method1"
The program does not compile.
The program compiles and runs, printing "method2"
The program does not compile.
In this problem, Derived1 and Derived2 are children of the Base class. If we take a look at this line:
Base* bp = new Derived();
We are assigning a new Derived class to a base pointer. This will compile. Think of the Base as a larger object because it is the parent, so copying a smaller object into a larger one is acceptable.
Now let's look at this one:
Derived2* d2p = bp;
This line will cause the program to not compile. Since the Base class is considered the "bigger" object, copying a bigger object into a "smaller" one will result in a failure to copy everything over, this is known as a Slicing Problem.
We don't even have to look at the next line because we know that the program wil crash.
Example Question #1 : Compile Time Errors
Given:
const int x = 10;
Which of the following will compile?
const int * r = &x
int * p = &x
int * const q = &x
All of the above
None of the above
const int * r = &x
First we take a look at the given statement:
const int x = 10;
the const in front of "int" means that x will always hold the value of 10 and it will not change.
Let's observe all the choices.
int *p =&x
This line says to assign the address of x (in memory) to the pointer p. This however, will not compile because int * p is not marked as const. x is marked as a const so this forces int * p to be a const as well.
int * const q = &x
There is a const in this case but it is in the wrong place
const int * r = &x
The const is in the correct place and this is the correct answer
Example Question #1 : Compile Time Errors
class Base{
protected:
void method();
};
class Derived : public Base{
};
int main(){
Base b;
b.method(); //Line A
Derived d;
d.method(); //Line B
}
Which of the following is true?
Line A will compile
Line B will compile
Line A will not compile
Line B will compile
Line A will not compile
Line B will not compile
None of these
Line A will compile
Line B will not compile
Line A will not compile
Line B will not compile
To understand this question, we have to understand what protected method means. A protected method is a method that is accessible to methods inside it's own class as well as it's children. This means that a protected method can be called in the child class.
We can see that method() is called inside main. This should already raise a red flag. A protected class is being called outside of the child class so it will not compile. Even those it's being called on the Base and Derived objects, the calls are not made inside Base and Derived class so neither line will compile.
Example Question #2 : Debugging
Consider the following code:
Object[] objects = new Object[20];
for(int i = 0; i < objects.length; i++) {
switch(i % 4) {
case 0:
objects[i] = new Integer(i + 3);
break;
case 1:
objects[i] = "This val: " + i;
break;
case 2:
objects[i] = new Double(i * 4.4);
break;
case 3:
objects[i] = "That val: " + (i*12);
break;
}
}
String s = (String)objects[8];
System.out.println(s);
What is the error in the code above?
There are no errors.
There will be a ClassCastException thrown.
There is an array overrun.
You cannot assign various types to the array in that manner.
There will be a NullPointerException.
There will be a ClassCastException thrown.
In order to understand the error in this code, you must understand what the loop is doing. It is assigning variable types to the array of Object
objects based upon the remainder of dividing the loop control variable i
by 4. You thus get a repeating pattern:
Integer, String, Double, String, Integer, String, Double, String, . . .
Now, index 8 will be an Integer (for it has a remainder of 0). This means that when you do the type cast to a String, you will receive a TypeCastException for the line reading:
String s = (String)objects[8];
Example Question #1 : Run Time Errors
public static int[][] doWork(int[][] a, int[][] b) {
int[][] ret = new int[a.length][a[0].length];
for(int i = 0; i < a.length; i++) {
for(int j = 0; j < a[i].length; j++) {
ret[i][j] = a[i][j] + b[i][j];
}
}
return ret;
}
In the code above, what is the potential error that will not be caught on the following line?
ret[i][j] = a[i][j] + b[i][j];
The array a
may be set to null
The arrays may contain null values
The array could go out of bounds
The arrays may not be initialized
The code is fine as it is written
The array could go out of bounds
At this point of the code, it is not possible for a
or b
to be null. Furthermore, these arrays cannot contain null values, for they are made up of primitive types (int
types). These cannot be set to null, as they are not objects. The potential error here is a little bit abstruse, but it is important to note. There is one possible error, namely that the 2D arrays are "ragged"; however we don't need to worry about this for the exam. Still, it is also possible that the array b
(presuming that it is not null) is not the same size as the array a
. Since we are using a
to set our loop counts, this could potentially mean that we overrun the array b
by using index values that are too large. (Note, it is also possible that b
could be null, causing an error; however, there are no answer choices for that possibility.)
All Computer Science Resources
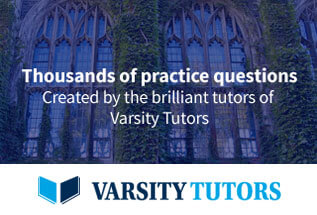