All Computer Science Resources
Example Questions
Example Question #1 : Testing
The function fun
is defined as follows:
public int fun(int[] a)
{
a[a.length - 1] = a[0];
return a[0] + (a[0] % 2);
}
a[0]
after the following code segment is executed?int[] a = {3, 6, 9, 12};
a[0] = fun(a);
12
3
6
9
13
12
The first part of fun
assigns the value of the last location of the array to the first location. Then, it returns the a[0] + (a[0] % 2)
. That last portion will be a "1
" if a[0]
is odd, and "0
" if a[0]
is even. Since a[0] == 12
, which is even, the expression evaluates to 12 + 0
, which is 12
.
Example Question #2 : Testing
x
, y
, and z
after the following code is executed?int x = 4, y = 3, z;
for (int i = 0; i < 5; i++)
{
z = x + y;
y = x - y;
x = z;
}
x == 14, y == 2, z == 14
x == 16, y == 12, z== 16
x == 28, y == 4, z== 28
x == 28, y == 4, z== 4
x == 32, y == 24, z == 32
x == 28, y == 4, z== 28
The loop will run 5 times. The values of x
, y
, and z
after each run will be as follows:
i == 0: x == 7, y == 1, z == 7
i == 1: x == 8, y == 6, z == 8
i == 2: x == 14, y == 2, z == 14
i == 3: x == 16, y == 12, z == 16
i == 4: x == 28, y == 4, z == 28
At the end, i
will equal 5, and the values will no longer change.
Example Question #3 : Program Analysis
if (s.length() <= 5)
return s5 + s4 + s3 + s2 + s1;
else
return s1 + s2 + mystery(s3) + s4 + s5;
}
What is the output of
System.out.println(mystery("ABNORMALITIES"));
None of these answers is correct.
SEITRMALIONBA
SEITILAMRONBA
ABNOILAMRTIES
ABNORMALITIES
ABNOILAMRTIES
The .substring()
method takes the character at the first number in the arguments, and goes through the String until it reaches the second number in the arguments, without copying the character at the second number.
In the first part of mystery()
, the Strings s1, s2, s3, s4, and s5 are made and filled. If n = # of characters in s, s1 gets the first character in s, s2 gets the second character in s, s3 gets the third through n-2 characters, s4 gets the n-1 character, and s5 gets the last character.
Let's look at the second portion of mystery().
if (s.length() <= 5)
return s5 + s4 + s3 + s2 + s1;
else
return s1 + s2 + mystery(s3) + s4 + s5;
The if
statement checks the length of s, and if it's less than or equal to 5, it returns a String made from s5, followed by s4, etc. If s were equal to "abcde", then the if
would evaluate to true, and would return "edcba". In recursion, this is known as the "base case".
The else
statement is for strings that are greater than 5 characters in length. It returns s1, followed by s2, then the result of mystery(s3), then s4 and s5. The fact that it calls itself makes this recursion.
Let's step through the example. The argument for mystery()
, s, is "ABNORMALITIES". After the first part, this is the result:
else
, so it returns the following:A + B + mystery(NORMALITI) + E + S
else
, so it returns the following:N + O + mystery(RMALI) + T + I
A + B + N + O + mystery(RMALI) + T + I + E + S
if
this time. It returns the following:I + L + A + M + R
A + B + N + O + I + L + A + M + R + T + I + E + S
Example Question #3 : Testing
Consider the Array
int[] arr = {1, 2, 3, 4, 5};
What are the values in arr
after the following code is executed?
for (int i = 0; i < arr.length - 2; i++)
{
int temp = arr[i];
arr[i] = arr[i+1];
arr[i+1] = temp;
}
OutOfBoundsException
15432
12345
23451
54321
23451
We start with an array, arr,
of size 5, containing {1, 2, 3, 4, 5}.
The loop in the code,
for (int i = 0; i < arr.length - 2; i++)
loops through the array up to the second to last cell, given that arr.length - 2
is the index of the second to last cell, and it starts at the first cell.
Let's look at the code inside the loop.
int temp = arr[i];
arr[i] = arr[i+1];
arr[i+1] = temp;
arr[0] == 1
arr[1] == 2
temp = 1
arr[0] = 2
arr[1] = 1
arr[] == {2, 1, 3, 4, 5}
arr[1] == 1
arr[2] == 3
temp = 1
arr[1] = 3
arr[2] = 1
arr[] == {2, 3, 1, 4, 5}
When i = 2
arr[2] == 1
arr[3] == 4
temp = 1
arr[2] = 4
arr[3] = 1
arr[] == {2, 3, 4, 1, 5}
arr[3] == 1
arr[4] == 3
temp = 1
arr[3] = 3
arr[4] = 1
arr[] == {2, 3, 4, 5, 1}
As the loop progresses, it moves whatever value is in arr[0]
all the way to the end of the array.
Example Question #1 : Identifying Boundary Cases
Identify a user error that could occur in this program
UserInput ui = new UserInput(); // input from the user
int s = (Integer)ui;
System.out.println(s);
The user input could not be an integer
The parseInt statement is incorrect
There is nothing wrong
The user can never do anything wrong
The user input could not be an integer
The user could input a string and the cast to an (Integer) would cause a runtime exception. If there is a runtime exception, the program will stop and open up vulnerabilities to hackers. Once a hacker knows how to halt a program, they can start input bad data to see a database schema to collect data. One way to fix this would be using a utility method such as parseInt(ui).
Example Question #1 : Compile Time Errors
Consider the following code:
public static class Rectangle {
private double width, height;
public Rectangle(double w,double h) {
width = w;
height = h;
}
public double getArea() {
return width * height;
}
public double getPerimeter() {
return 2 * width + 2 * height;
}
}
public static class Square extends Rectangle {
public Square(double s) {
super(s,s);
}
}
public static void main(String[] args) {
Rectangle[] rects = new Rectangle[6];
for(int i = 0; i < 6; i++) {
if(i % 2 == 0) {
rects[i] = new Rectangle(i+10,i + 20);
} else {
rects[i] = new Square(i+20);
}
}
Square s = rects[1];
}
What is the error in the code above?
You need to use "implements", not "extends" for the class Square
.
There is an error in the declaration of the Rectangle
array.
The final assignment operation cannot be done.
There is an array overrun.
You cannot assign a Square
object to an array of Rectangle
objects.
The final assignment operation cannot be done.
This code fills up the 6 member array with alternating Rectangle and Square objects. You can do this because the Square class is a subclass of Rectangle. That is, since Squares are Rectangles, you can store Square objects in Rectangle variables. However, even though rects[1]
is a square, you CANNOT immediately reassign that to a Square object. The code has now come to consider all of the objects in the array as being Rectangle objects. You would need to explicitly type cast this to get the line to work:
Square s = (Square)(rects[1]);
Example Question #1 : Program Analysis
What is the error in the following code?
int val1 = -14,val2 = 4;
final int val3 = 9;
double val4 = 4.1;
double val5 = 3.1;
val1 = val2 * val3;
val3 = val1 * 12;
val5 = val1 - val3;
val4 = val2 + val5;
You must define val2
on its own line.
You cannot assign the result of an integer subtraction to val5
.
You cannot multiply val3
by val2
.
You cannot assign the new integer value to val3
.
You cannot perform mixed addition or subtraction with doubles and integers.
You cannot assign the new integer value to val3
.
The only error among the options given is the fact that this code assigns a new value to the variable val3
, which is defined as a constant. (This is indicated by the keyword final
before the rest of its declaration.) You cannot alter constants once they have been declared. Thus, the following line will cause a compile-time error:
val3 = val1 * 12;
Example Question #1 : Debugging
public static void main(String[] args) {
int[] x = {3,4,4,5,17,4,3,1};
int[] y = remove(x,4);
for(int i = 0; i < y.length; i++) {
System.out.print(y[i] + " ");
}
}
public static boolean remove(int[] arr, int val) {
boolean found = false;
int i;
for(i = 0; i < arr.length && !found; i++) {
if(arr[i] == val) {
found = true;
}
}
if(found) {
for(int j = i; j < arr.length;j++) {
arr[j - 1] = arr[j];
}
arr[arr.length - 1] = 0;
}
return found;
}
What is the error in the code above?
In the remove
method, there is a variable that has not been initialized before being used
There is no error in the code
There are multiple options to delete, which is not supported by the function
There is a type mismatch in one of the assignments
The output loop in main
will overrun the array y
There is a type mismatch in one of the assignments
The problematic line is this one:
int[] y = remove(x,4);
Notice that the variable y is defined as an array. Now, it is tempting to think (without looking too closely) that the remove
method returns the array after the removal has been accomplished; however, this is not how the logic works in the remove
method. Instead, it returns a boolean indicating whether or not this removal was successful or not (i.e. it tells you whether or not it actually found the value). Therefore, you cannot make an assignment like the one above, for the two types are not the same. That is, y
is an integer array, while remove
returns a boolean value!
Example Question #2 : Debugging
public interface ServerInstance {
byte[] readBytes();
boolean writeBytes(byte[]b);
boolean wake();
boolean status();
void sleep();
}
public class MyHost implements ServerInstance {
boolean running = false;
public boolean wake() {
// Other logic code here...
return running;
}
public boolean status() {
// Other logic code here...
return running;
}
public byte[] readBytes() {
byte[] buffer = null;
// Other logic code here...
return buffer;
}
public void sleep() {
// Other logic code here...
running = false;
}
public byte[] writeBytes(byte[] b) {
// Other logic code here...
return b;
}
// Other methods...
}
What is the error in the code above?
The writeBytes
method is not properly defined
There is no error
The readBytes
method initializes the variable buffer
to null
The sleep
method does not return anything
The word extends
should be used, not implements
The writeBytes
method is not properly defined
When you implement an interface, all of the methods defined in that interface must be written in the class that is proposing to be such an implementation. (Or, if they are not implemented there, you need to involve abstract classes—but that is not our concern here.) The methods must match the prototypes proposed in the interface. In the example code, ServerInstance
has a method writeBytes
that returns a boolean value. However, the MyHost
class has implemented this method as returning a byte[]
value. Since you cannot have different types of return values for methods with the same parameter set, Java interprets this as being the proposed implementation for writeBytes(byte[] b)
, and this method must return a boolean if MyHost
is to implement ServerInstance
.
Example Question #2 : Compile Time Errors
public static void foo() {
int x = 10; y = 21, z = 30;
int[] arr = null;
for(int i = 0; i < y; i+= 4) {
arr = new int[i / 5];
}
for(int i = 0; i < x; i++) {
arr[i] = z / i;
}
for(int i = 0; i < z; i++) {
arr[i] = z * i;
}
}
Which of the following lines of code will cause a compile-time
error?
arr = new int[i / 5];
arr[i] = z / i;
None of these lines will cause a compile-time
error
int x = 10; y = 21, z = 30;
arr[i] = z * i;
int x = 10; y = 21, z = 30;
An error in compilation occurs before any code even attempts to execute. Thus, it is primarily a syntactical error in the code. In the selection above, the line
int x = 10; y = 21, z = 30;
has a semicolon right after 10.
This causes an error in the code directly following on this, for the code
y = 21, z = 30;
is not valid Java code.
There are other errors in this code. arr[i] = z / i;
will cause a divide by 0 error when i is 0. Also, arr[i] = z * i;
will overrun the bounds of the array arr
. However, these are not compile-time errors—i.e. errors that occur before the code is even able to run!
Certified Tutor
All Computer Science Resources
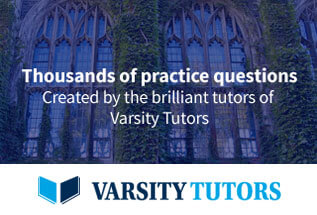