All Computer Science Resources
Example Questions
Example Question #1 : Run Time Errors
Consider the following code:
Object[] objects = new Object[20];
for(int i = 0; i < objects.length; i++) {
switch(i % 4) {
case 0:
objects[i] = new Integer(i + 3);
break;
case 1:
objects[i] = "This val: " + i;
break;
case 2:
objects[i] = new Double(i * 4.4);
break;
case 3:
objects[i] = "That val: " + (i*12);
break;
}
}
String s = (String)objects[8];
System.out.println(s);
What is the error in the code above?
There is an array overrun.
You cannot assign various types to the array in that manner.
There are no errors.
There will be a NullPointerException.
There will be a ClassCastException thrown.
There will be a ClassCastException thrown.
In order to understand the error in this code, you must understand what the loop is doing. It is assigning variable types to the array of Object
objects based upon the remainder of dividing the loop control variable i
by 4. You thus get a repeating pattern:
Integer, String, Double, String, Integer, String, Double, String, . . .
Now, index 8 will be an Integer (for it has a remainder of 0). This means that when you do the type cast to a String, you will receive a TypeCastException for the line reading:
String s = (String)objects[8];
Example Question #2 : Run Time Errors
public static int[][] doWork(int[][] a, int[][] b) {
int[][] ret = new int[a.length][a[0].length];
for(int i = 0; i < a.length; i++) {
for(int j = 0; j < a[i].length; j++) {
ret[i][j] = a[i][j] + b[i][j];
}
}
return ret;
}
In the code above, what is the potential error that will not be caught on the following line?
ret[i][j] = a[i][j] + b[i][j];
The array could go out of bounds
The code is fine as it is written
The arrays may contain null values
The array a
may be set to null
The arrays may not be initialized
The array could go out of bounds
At this point of the code, it is not possible for a
or b
to be null. Furthermore, these arrays cannot contain null values, for they are made up of primitive types (int
types). These cannot be set to null, as they are not objects. The potential error here is a little bit abstruse, but it is important to note. There is one possible error, namely that the 2D arrays are "ragged"; however we don't need to worry about this for the exam. Still, it is also possible that the array b
(presuming that it is not null) is not the same size as the array a
. Since we are using a
to set our loop counts, this could potentially mean that we overrun the array b
by using index values that are too large. (Note, it is also possible that b
could be null, causing an error; however, there are no answer choices for that possibility.)
Example Question #11 : Program Analysis
Consider the following code:
public static int[] del(int[] a,int delIndex) {
if(delIndex < 0 || delIndex >= a.length) {
return null;
}
int[] ret = new int[a.length - 1];
for(int i = 0; i < a.length; i++) {
if(i != delIndex) {
ret[i] = a[i];
}
}
return ret;
}
What is the error in the code above?
The use of the array index i is incorrect.
You need to implement a swap for the values.
The intial conditional (i.e. the if statement) has incorrect logic.
The loop is infinite.
There is a null pointer exception.
The use of the array index i is incorrect.
The problematic line in the code above is the one that reads:
ret[i] = a[i];
This is going to work well until the end of the array. The variable i is going to go for the length of the array a. However, the array ret is one less in length. This means that you will overrun your array, getting an ArrayIndexOutOfBoundsException at the very end of the looping. You would need to implement two indices—one of which will not be incremented on that one time when you skip the element that is removed from the array.
Example Question #11 : Debugging
What is wrong with this loop?
int i = 0;
int count = 20;
int[] arr = [1, 3, 4, 6, 8, 768, 78, 9]
while (i < count) {
if (i > 0) {
arr[i] = count;
}
if (i < 15) {
System.out.println("HEY");
}
}
The loop is infinite
if statement is incorrect
count never gets decremented
it prints Hey too many times
The loop is infinite
The loop is infinite because i never gets incremented to become greater than count.
The loop in this case is,
while (i < count) {
if (i > 0) {
arr[i] = count;
}
and the error occurs because there is no terminating factor in the while loop. A terminating factor would be,
while(i<count){
if(i>0, i<10, i++){
arr[i] = count;
}
Example Question #1 : Identifying & Correcting Errors
Consider the following code:
int num = 1;
for(int i = 0; i < 10; i++) {
num *= i;
}
The code above is intended to provide the continuous product from num to 10. (That is, it provides the factorial value of 10!.) What is the error in the code?
You cannot use *= in a loop.
The loop control is incorrect.
The loop control, the i initialization, and the initialization for num are all wrong.
Both the i intialization and the loop control value are incorrect.
The i initialization is wrong.
Both the i intialization and the loop control value are incorrect.
This loop will indeed run 10 times. However, notice that it begins on 0 and ends on 10. Thus, it will begin by executing:
num *= 0
Which is the same as:
num = num * 0 = 0
Thus, you need to start on 1 for i. However, notice also that you need to go from 1 to 10 inclusive. Therefore, you need to change i < 10 to i <= 10.
Example Question #21 : Program Analysis
Consider the code below:
public class Clock {
private int seconds;
public Clock(int s) {
seconds = s;
}
public void setTime(int s) {
seconds = s;
}
public void setSeconds(int s) {
int hoursMinutes = seconds - seconds % 60;
seconds = hoursMinutes + s;
}
public void setMinutes(int min) {
int hours = seconds / 3600;
int currentSeconds = seconds % 60;
seconds = hours + min * 60 + currentSeconds;
}
}
Which of the following issues could be raised regarding the code?
There is insufficient data verification for the mutator methods.
The setMinutes
method should return an integer value.
There are no issues.
There is an error in the setMinutes
calculation.
There is a potential null pointer exception in one of the methods.
There is insufficient data verification for the mutator methods.
Recall that a "mutator" is a method that allows you to change the value of fields in a class. Now, for the setTime
, setMinutes
, and setSeconds
methods, you do not check several cases of errors. For instance, you could give negative values to all of these methods without causing any errors to be noted. Likewise, you could assign a minute or second value that is greater than 60, which could cause errors as well. Finally, you could even make the clock have a value that is greater than "23:59:59", which does not make much sense at all!
Example Question #12 : Debugging
What is wrong with the following code?
- int main()
- {
- bool logic;
- double sum=0;
- for(j=0;j<3;j++)
- {
- sum=sum+j;
- }
- if (sum>10)
- {
- logic=1;
- }
- else
- {
- logic=0;
- }
- }
- }
The code has no errors.
Variable j is not defined.
There is an opening/closing bracket missing.
You cannot define the variable logic as a bool.
You must define the sum variable as an integer, not a double.
Variable j is not defined.
If you notice, in our for loop, the integer j is used as the iteration variable. However, no where in the code is that variable defined. To fix this issue this could have been done.
for (int j=0;j<3,j++)
Note the bold here is inserted. We need to define j here. We could have also defined j as a double.
Example Question #1 : Identifying & Correcting Errors
FILE INPUT/OUTPUT
Consider the following C++ code:
1. #include <iostream>
2. #include <fstream>
3. using namespace std;
4. int main() {
5. ifstream outputFile;
6. inputFile.open("TestFile.txt");
7. outputFile << "I am writing to a file right now." << endl;
8. outputFile.close();
9. //outputFile << "I'm writting on the file again" << endl;
10. return 0;
11. }
What is wrong with the code?
The ifstream library should be included.
Testfile.txt shouldn't have double quotations around it.
There is nothing wrong with the code.
The outputFile should be of type "ofstream", not "ifstream".
The outputFile should be of type "ofstream", not "ifstream".
Type ifstream objects are used to read from files, NOT to write to a file. To write to a file you can use ofstream. You can also you fstream to both read and write to and from a file.
Example Question #2 : Identifying & Correcting Errors
a. public void draw() {
b. int i = 0;
c. while(i < 15){
d. system.out.println(i);
e. i++
f. }
g. }
Which lines of code have errors?
cb
e
d c
b
d e
d e
line d's error is that system.out.println (i); is not capitalized.
line e's error is that there is a missing semicolon.
All Computer Science Resources
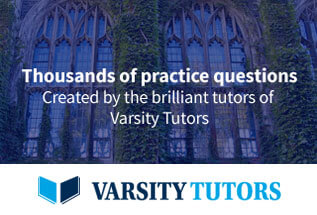