All Computer Science Resources
Example Questions
Example Question #1 : Top Down Development
Consider the following code:
int[] vals = {6,1,41,5,1};
int[][] newVals = new int[vals.length][];
for(int i = 0; i < vals.length; i++) {
newVals[i] = new int[vals[i]];
for(int j = 0; j < vals[i];j++) {
newVals[i][j] = vals[i] * (j+1);
}
}
What does the code above do?
It creates a 2D matrix with the vals array for every row.
It sums the values in vals, placing the outcome in newVals.
It performs a matrix multiplication on vals and newVals, storing the final result in newVals.
The code creates a 2D array that contains all multiples from 1 to 5 for the members of vals.
The code creates a 2D array that contains the multiples for the members of vals, using each of those values to determine the number of multiples to be computed.
The code creates a 2D array that contains the multiples for the members of vals, using each of those values to determine the number of multiples to be computed.
Let's look at the main loop in this program:
for(int i = 0; i < vals.length; i++) {
newVals[i] = new int[vals[i]];
for(int j = 0; j < vals[i];j++) {
newVals[i][j] = vals[i] * (j+1);
}
}
The first loop clearly runs through the vals array for the number of items in that array. After this, it creates in the newVals 2D array a second dimension that is as long as the value in the input array vals. Thus, for 41, it creates a 3rd row in newVals that is 41 columns wide.
Then, we go to the second loop. This one iterates from 0 to the value stored in the current location in vals. It places in the given 2D array location the multiple of the value. Think of j + 1. This will go from 1 to 41 for the case of 41 (and likewise for all the values). Thus, you create a 2D array with all the multiples of the initial vals array.
Certified Tutor
Certified Tutor
All Computer Science Resources
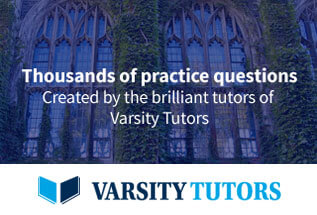