All Computer Science Resources
Example Questions
Example Question #1 : Top Down Development
Consider the following code:
int[] vals = {6,1,41,5,1};
int[][] newVals = new int[vals.length][];
for(int i = 0; i < vals.length; i++) {
newVals[i] = new int[vals[i]];
for(int j = 0; j < vals[i];j++) {
newVals[i][j] = vals[i] * (j+1);
}
}
What does the code above do?
It creates a 2D matrix with the vals array for every row.
It sums the values in vals, placing the outcome in newVals.
It performs a matrix multiplication on vals and newVals, storing the final result in newVals.
The code creates a 2D array that contains all multiples from 1 to 5 for the members of vals.
The code creates a 2D array that contains the multiples for the members of vals, using each of those values to determine the number of multiples to be computed.
The code creates a 2D array that contains the multiples for the members of vals, using each of those values to determine the number of multiples to be computed.
Let's look at the main loop in this program:
for(int i = 0; i < vals.length; i++) {
newVals[i] = new int[vals[i]];
for(int j = 0; j < vals[i];j++) {
newVals[i][j] = vals[i] * (j+1);
}
}
The first loop clearly runs through the vals array for the number of items in that array. After this, it creates in the newVals 2D array a second dimension that is as long as the value in the input array vals. Thus, for 41, it creates a 3rd row in newVals that is 41 columns wide.
Then, we go to the second loop. This one iterates from 0 to the value stored in the current location in vals. It places in the given 2D array location the multiple of the value. Think of j + 1. This will go from 1 to 41 for the case of 41 (and likewise for all the values). Thus, you create a 2D array with all the multiples of the initial vals array.
Example Question #1 : Object Oriented Development
Consider the following C++ pseudocode:
Class Car {
const int wheels = 4;
float milesPerGallon;
string make;
string model;
}
Car sportscar = new Car;
What is the difference between the class car
, and the object sportscar
?
sportscar
is instantiated from Car.
They are both instantiated from something else.
Car
is instantiated from sportscar
.
They are the same.
They have no relation.
sportscar
is instantiated from Car.
When programming within the Object-Oriented paradigm, think of the class as a blueprint, and the object as a house built from that blueprint.
The class Car
is a abstract specification that does not refer to any one particular instance. It is merely a protocol that all objects wishing to be cars should follow. The sportscar object is a realization of the car blueprint. It is a specific instance. In programming jargon, "sportscar
is instantiated from Car
."
Example Question #1 : Object Oriented Development
JAVA EXPLICIT TYPE CASTING
Consider the following JAVA code:
public static void main(String[] args)
{
double number1 = 99.05;
int number2 = (int) number1;
System.out.println("number1 = " + number1);
System.out.println("number2 = " + number2);
}
What would be the console output?
number1 = 99
number2 = 99
number1 = 99.05
number2 = 99
number1 = 99.05
number2 = 99.05
number1 = 99.05
number2 = 99.00
number1 = 99.05
number2 = 99
Type casting deals with assigning the value of a variable to another variable that is of a different type. In this case we have two variables one that is a double, and another that is an integer. number1 is a double that has a value of 99.05. However, when number2 is assigned the value of number1, there is some explicit type casting going on. When an integer is assigned the value of a double, it drops off the decimal places. This means that number2 has a value of 99.
Example Question #231 : Computer Science
Class Person {
int height;
float weight;
public:
int getHeight();
void setHeight(int);
float getWeight();
void setWeight(float);
};
What is the access level of height
and weight
?
friend
protected
virtual
private
public
private
Until an access specifier is given, all class members are implicitly private. All members defined after an access specifier is used will will have that access level until another access specifier is explicitly invoked. Since no access specifier was used, weight
and height
are automatically private.
Note that virtual
is not an access keyword.
Example Question #4 : Implementation Techniques
Consider the following code:
public static class Clock {
private int seconds;
public Clock(int s) {
seconds = s;
}
public void setTime(int s) {
seconds = s;
}
public void setSeconds(int s) {
int hoursMinutes = seconds - seconds % 60;
seconds = hoursMinutes + s;
}
public void setMinutes(int min) {
int hours = seconds / 3600;
int currentSeconds = seconds % 60;
seconds = hours + min * 60 + currentSeconds;
}
}
Which of the following represents a method that returns the minute value of the clock?
public int getMinutes() {
int hours = seconds / 3600;
return (seconds - hours * 3600);
}
public int getMinutes() {
int hours = seconds / 3600;
return (seconds - hours * 3600) / 60;
}
public int getMinutes() {
return seconds / 60;
}
public int getMinutes() {
return mins;
}
public int getMinutes() {
return seconds * 60;
}
public int getMinutes() {
int hours = seconds / 3600;
return (seconds - hours * 3600) / 60;
}
This clock class is defined as having only seconds in its fields, so you have to convert this value for any of the accessors and mutators. Therefore, you need to perform careful (though simple) mathematics for this conversion. To calculate the number of minutes in a given number of seconds, you first need to remove the hours from total count. First, compute the hours using integer division (which will drop the fractional portion of the decimal). This is:
int hours = seconds / 3600;
Next, you need to figure out how many seconds are in that value of hours. This is helpful precisely because integer division drops the decimal portion. Thus, you will subtract off:
hours * 3600
from the total seconds.
This gives you the seconds that apply to the minutes and seconds of the time. Therefore, you will finally need to divide by 60 to isolate the minutes.
Example Question #71 : Program Implementation
Consider the following code:
public class Clock {
private int seconds;
public Clock(int s) {
seconds = s;
}
public void setTime(int s) {
seconds = s;
}
public void setSeconds(int s) {
int hoursMinutes = seconds - seconds % 60;
seconds = hoursMinutes + s;
}
public void setMinutes(int min) {
int hours = seconds / 3600;
int currentSeconds = seconds % 60;
seconds = hours + min * 60 + currentSeconds;
}
}
Which of the following defines a toString method that will output the time in 24-hour format in the following form:
1:51:03
(Notice that you need to pad the minutes and seconds. You can call 12 midnight "0".)
public String toString() {
int hours = seconds / 3600;
int mins = (seconds - hours * 3600);
String minString = ""+mins;
if(mins < 10) {
minString = "0" + minString;
}
int secs = seconds % 60;
String secString = "" + secs;
if(secs < 10) {
secString = "0" + secString;
}
return hours + ":"+minString+":"+secString;
}
public String toString() {
int hours = seconds / 3600;
int mins = seconds - (hours * 3600) / 60;
String minString = ""+mins;
if(mins < 10) {
minString = "0" + minString;
}
int secs = seconds * 60;
String secString = "" + secs;
if(secs < 10) {
secString = "0" + secString;
}
return hours + ":"+minString+":"+secString;
}
public String toString() {
int hours = seconds / 3600;
int mins = (seconds - hours * 3600) / 60;
String minString = ""+mins;
if(mins < 10) {
minString = "0" + minString;
}
int secs = seconds % 60;
String secString = "" + secs;
if(secs < 10) {
secString = "0" + secString;
}
return hours + ":"+minString+":"+secString;
}
public String toString() {
int hours = seconds / 60;
int mins = seconds - (hours * 3600) / 60;
String minString = ""+mins;
if(mins < 10) {
minString = "0" + minString;
}
int secs = seconds * 60;
String secString = "" + secs;
if(secs < 10) {
secString = "0" + secString;
}
return hours + ":"+minString+":"+secString;
}
public String toString() {
int hours = seconds / 3600;
int mins = (seconds - hours * 3600) / 60;
String minString = ""+mins;
if(mins < 10) {
minString += "0";
}
int secs = seconds % 60;
String secString = "" + secs;
if(secs < 10) {
secString += "0";
}
return hours + ":"+minString+":"+secString;
}
public String toString() {
int hours = seconds / 3600;
int mins = (seconds - hours * 3600) / 60;
String minString = ""+mins;
if(mins < 10) {
minString = "0" + minString;
}
int secs = seconds % 60;
String secString = "" + secs;
if(secs < 10) {
secString = "0" + secString;
}
return hours + ":"+minString+":"+secString;
}
There are several points to be noted in this code. First, you need to calculate each of the constituent parts from the seconds stored in the class. The "display value" of seconds is relatively easy. This is just the remainder of a division by 60. Consider:
61 seconds => This is really 1 minute and 1 second.
155 seconds => This is really 2 minutes and 35 seconds.
So, you know that the display seconds are:
seconds % 60
You must compute the hours using integer division (which will drop the fractional portion of the decimal). This is:
int hours = seconds / 3600;
Next, you need to figure out how many seconds are in that value of hours. This is helpful precisely because integer division drops the decimal portion. Thus, you will subtract off:
hours * 3600
from the total seconds.
This gives you the seconds that apply to the minutes and seconds of the time. Therefore, you will finally need to divide by 60 to isolate the minutes.
Then, you must pad the values. This is not done using +=
, which would add the "0" character to the end of the string. Instead, it is done by the form:
secString = "0" + secString;
Example Question #232 : Computer Science
Given a vector of ints called "intVec", write a "ranged for" loop, also sometimes known as a "for each" loop, to double the values fof all elements in a vector. (In C++)
for( int i = 0; i < intVec.size(); ++i){
intVec[i] *= 2;
}
for( int& i : intVec(){
i * = 2;
}
for( int& i : intVec(){
i * 2;
}
for( int i = 0; i < intVec.size(); ++i){
intVec[i] * 2;
}
for( int i : intVec(){
i * = 2;
}
for( int& i : intVec(){
i * = 2;
}
A "for each" loop was introduced in C++ 11 which allows the user to loops through a container of items without writing a traditional loop. Let's take a look at all the choices.
for( int i : intVec(){
i * = 2;
}
Although this looks like the correct answer, it's not. The int i in the first part of the for loop is passed in "by value", which means that a copy of intVec will be made and the integers inside the copy will be doubled. The integers inside the original intVec vector will be unaffected. To fix this problem, int i needs to be passed into the for loop "by reference", which is done by adding a "&" symbol after "int".
The correct loop is:
for( int& i : intVec(){
i * = 2;
}
Let's take a look at the other choices:
for( int& i : intVec(){
i = 2;
}
This one will not work because it is setting all items in intVec to 2, not doubling them.
All the other for loops are not "for each" loops so they are incorrect even if they accomplish the same output.
All Computer Science Resources
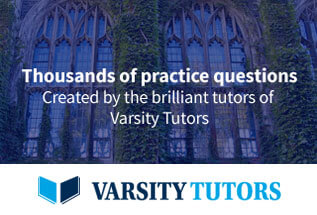