All Computer Science Resources
Example Questions
Example Question #31 : Programming Constructs
Create the method stub for a void method called ingredients that takes in spaghetti, sauce, and meatballs as parameters where the parameters are all strings.
void ingredients(String spaghetti, String sauce, String meatballs);
ingredients(spaghetti, sauce, meatballs);
void ingredients(spaghetti, sauce, meatballs);
ingredients(String spaghetti, String sauce, String meatballs);
void ingredients(String spaghetti, String sauce, String meatballs);
The answer choice is correct because it defines a void method (void ingredients) that takes in the three string parameters (String spaghetti, String sauce, String meatballs). The other answer choices are missing either the void type on the method stub or the type of parameter. Note both the return type of the method (void in this example) and the types of the parameters are very important.
Example Question #1 : Parameter Declarations
What keyword is needed to create a class variable?
private
abstract
static
final
static
The static modifier makes the variable visible to the entire class, including the various methods in the program. Final just means that variable shall not be changed through out the code. Private just changes visibility not accessibility. For a variable to be abstract, the method itself would need to abstract and that doesn't really influence accessibility.
Example Question #32 : Program Implementation
Which of the following blocks of code will output an evenly-spaced 2D array (i.e. an evenly-spaced matrix)? For example, a simple evenly-spaced matrix is:
1 2 3
4 5 6
7 8 9
int[][] matrix = {{134,135,12},{31,1,41},{-1441,14,51},{14,5,134}};
for(int i = 0; i < matrix.length; i++) {
for(int j = 0; j < matrix[i].length; j++) {
System.out.print(matrix[i][j]);
}
System.out.print(" ");
}
int[][] matrix = {{134,135,12},{31,1,41},{-14441,14,51},{14,5,134}};
for(int i = 0; i < matrix.length; i++) {
for(int j = 0; j < matrix[i].length; j++) {
if(j != 0) {
System.out.print("\t\t");
}
System.out.print(matrix[i][j]);
}
System.out.println();
}
int[][] matrix = {{134,135,12},{31,1,41},{-14441,14,51},{14,5,134}};
for(int i = 0; i < matrix.length; i++) {
for(int j = 0; j < matrix[i].length; j++) {
if(j != 0) {
System.out.print(" ");
}
System.out.println(matrix[i][j]);
}
System.out.println();
}
int[][] matrix = {{134,135,12},{31,1,41},{-14441,14,51},{14,5,134}};
for(int i = 0; i < matrix.length; i++) {
for(int j = 0; j < matrix[i].length; j++) {
if(j == 0) {
System.out.print("\t\t");
}
System.out.print(matrix[i][j]);
}
System.out.println();
}
int[][] matrix = {{134,135,12},{31,1,41},{-14441,14,51},{14,5,134}};
for(int i = 0; i < matrix.length; i++) {
for(int j = 0; j < matrix[i].length; j++) {
if(j != 0) {
System.out.print(" ");
}
System.out.print(matrix[i][j]);
}
System.out.println();
}
int[][] matrix = {{134,135,12},{31,1,41},{-14441,14,51},{14,5,134}};
for(int i = 0; i < matrix.length; i++) {
for(int j = 0; j < matrix[i].length; j++) {
if(j != 0) {
System.out.print("\t\t");
}
System.out.print(matrix[i][j]);
}
System.out.println();
}
There are several critical points to look at in the code given as possible answers above.
First, you need to make sure that the main output is not a new line. Thus, the following is incorrect:
System.out.println(matrix[i][j]);
This would create a new line for every single value!
Next, you need to make sure that a new line is output after every row of the matrix. This is the final output statement. Thus, it cannot be:
System.out.print(" ");
This would only output a character with no new line.
Finally, for everything except for the first element of each row, you will need to output sufficient padding before the given element element (so as to make the matrix to be evenly-spaced). We are not going to be too particular here about the problem of very big numbers—the values are given to us as is. Note that it is insufficient to use a mere space for this. You should use a tab character (defined as '\t'). A space will not guarantee enough spacing (no pun intended)!
The correct answer has the following logic:
if(j != 0) {
System.out.print("\t\t");
}
This means "when the column is not the first column" (i.e. j != 0), "then output tabs to space sufficiently."
Example Question #1 : Console Output
Consider the following code:
for(int i = 1; i <= 10; i++) {
for(int j = 0; j < (20 - i * 2) / 2; j++) {
System.out.print(" ");
}
for(int j = 0; j < i * 2; j++) {
System.out.print("*");
}
for(int j = 0; j < (20 - i * 2) / 2; j++) {
System.out.print(" ");
}
System.out.println();
}
Describe the output of the code above.
An arrow pointing upward, having a base 20 characters wide and a top that is 2 characters wide.
An arrow pointing downward, having a base 20 characters wide and a top that is 2 characters wide.
A left-facing arrow with a large base of 20 characters in size and a point that is 1 character wide.
A right-facing arrow with large base of 20 characters in size and a point that is 2 characters wide.
An arrow pointing upward, having a base 20 characters wide and a top that is 1 character wide.
An arrow pointing upward, having a base 20 characters wide and a top that is 2 characters wide.
This code is best explained by analyzing it directly. Comments will be provided in bold below:
// The loop runs from 1 to 10
for(int i = 1; i <= 10; i++) {
// First, you run through 0 to some number
// That number is computed by taking the current value of i, doubling it,
// subtracting that from 20, then dividing by 2.
// So, consider the following values for i:
// 0: 20 - 0 = 20; Divided by 2: 10
// 1: 20 - 2 = 18; Divided by 2: 9
// 2: 20 - 2 = 16; Divided by 2: 8
// etc...
// Thus, you will output single spaces that number of times (10, 9, 8...)
for(int j = 0; j < (20 - i * 2) / 2; j++) {
System.out.print(" ");
}
// Next, you will output an asterix i * 2 number of times. Thus, your
// smallest value is 2 and your largest 20.
for(int j = 0; j < i * 2; j++) {
System.out.print("*");
}
// This is just a repeat of the same loop from above
for(int j = 0; j < (20 - i * 2) / 2; j++) {
System.out.print(" ");
}
// This bumps us down to the next line
System.out.println();
}
Thus, you have output that basically has even padding on the left and right, with an increasing number of asterix characters (2, 4, 6, etc). This is an upward-facing arrow, having a point of width 2 and a base of width 20.
Example Question #2 : Console Output
Consider the following code:
What is the output of the method call mystery("Green eggs and ham")
,G,r,e,e,n, ,e,g,g,s, ,a,n,d, ,h,a,m,
Green,eggs,and,ham,
Green eggs and ham,
Greeneggsandham,
ham,
,G,r,e,e,n, ,e,g,g,s, ,a,n,d, ,h,a,m,
The method String.split() splits a String into an array of Strings separated according to the expression within the method argument. The expression String.split("") splits the String at every character. The "for" loop concatenates the elements of the String array together, separated by a comma.
Example Question #4 : Console Output
public static void main(String[] args) {
int[][] x = {{4,5,6,7},{91,15,14,13}};
int[][] y = {{-13,4,41,14},{14,5,13,3}};
int[][] z = doWork(x,y);
for(int i = 0; i < z.length; i++) {
for(int j = 0; j < z[0].length;j++) {
System.out.print(z[i][j] + " ");
}
System.out.println();
}
}
public static int[][] doWork(int[][] a, int[][] b) {
int[][] ret = new int[a.length][a[0].length];
for(int i = 0; i < a.length; i++) {
for(int j = 0; j < a[i].length; j++) {
ret[i][j] = a[i][j] + b[i][j];
}
}
return ret;
}
What is the console output for the code above?
236
-9 9 47 21
105 20 27 16
22 133
46 35
4 4 6 7
14 5 13 3
68
168
-9 9 47 21
105 20 27 16
The doWork
method implements a relatively standard type of 2D array iteration, one that goes through each element. The outer loop goes through the first dimension of the array. Then, the inner loop provides index for the second dimension. From this, you get the total 2D index [i][j]
. The line ret[i][j] = a[i][j] + b[i][j];
actually performs the operation at the given index. Here, it performs an addition, combining the values at [i][j]
found in the two arrays. This gives you the sum at each index of ret
. The main method outputs each of these values, following the same standard algorithm for 2D array traversal.
Example Question #2 : Console Output
int a = 10, b = 5;
for(int i = 0; i < a; i+=2) {
for(int j = 0; j < i % b; j++) {
System.out.print("* ");
}
System.out.println();
}
What will be the console output for the code above?
* *
* * *
*
*
* *
* * *
* *
*
* *
* * *
*
* *
* * *
* * * *
* * * * *
* * * *
* * *
* *
*
* *
* * * *
*
* * *
* * *
* * *
* *
* *
* *
* * * *
*
* * *
All this problem requires is careful attention to the loop control variables. Notice that the first loop adds 2 to i every looping. This means that it will run for 0,2,4,6, and 8. Thus, a total of five lines will be printed.
Now, the second loop dictates the number of * characters that will be output per line. This is going to be based upon the result of the modulus i % b. Remember, modulus is a remainder calculation. Thus, you will have:
0 % 5 = 0
2 % 5 = 2
4 % 5 = 4
6 % 5 = 1
8 % 5 = 3
Hence, you will have the following (note the first line is empty, given the result of 0 % 5):
* *
* * * *
*
* * *
Example Question #1 : Console Output
What will the following code result in?
int main(){
int i = 7;
const int * ip = &i;
cout<< *ip << endl;
}
Compilation error
Runtime Error
None of the above.
8
7
7
Let's take a look at the code.
int i = 7;
This line assigns 7 to the variable "i".
const int * ip = &i;
This line creates a constant int pointer and assigns the address of "i" to the pointer.
cout << *ip << endl;
This line prints the dereference of the pointer, which holds the value 7.
Example Question #2 : Console Output
class Base{
public:
void foo(int n) { cout << "Base::foo(int)\n"; }
};
class Derive: public Base{
public:
void foo(double n) { cout << "Derived::foo(double)\n";}
};
int main(){
Derived der;
der.foo(42);
}
The above code is written in C++, what is the output of the program?
Base::foo(int).
The program does not compile.
None of the above.
Derived::foo(double).
The program does not generate any output.
Derived::foo(double).
This is an example of inheritance. The child class (Derived) is an inherited class of the parent class (Base). When the Derived object is created and the "foo" method is called, foo in the Derived class will be called. If there is the same method in the parent class and child class, the child's method will be called.
Example Question #32 : Programming Constructs
LOGIC WITH 2-D ARRAYS
Given:
int[][] myArray = { {1, 2},
{3, 4} };
What would the following statement print out to the console?
System.out.print(myArray[1][1] + 10);
14
11
12
13
14
Before anything is printed out into the console, the following is first evaluated:
myArray[1][1] + 10
myArray[1][1] is referring to the item that is in row=1 and column=1 of myArray. Taking note that arrays start with row 0 and column 0, we see that the item in row 1 column 1 is the number 4. Now we have the following: 4+10. This evaluates to 14. Therefore, the Java print statement will print out the number 14 on the console.
Certified Tutor
All Computer Science Resources
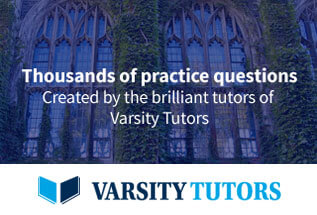