All Computer Science Resources
Example Questions
Example Question #21 : Programming Constructs
Why is the "default" keyword used in a switch statement?
It defaults the case statement the case 1 if the function is stuck in an infinite loop.
Default statements are unnecessary in case statements.
It makes the function default to a backup function if the case isn't present.
It sets a variable to be a default value in case that value isn't defined in the case statement.
To catch any remaining possible values that may not be included in the case statements.
To catch any remaining possible values that may not be included in the case statements.
The default keyword is used to catch any remaining cases not specified. For example, if you only want to check for certain hour of the day, say 2,3, and 4 o'clock, the default case would catch all the other hours. You could then create a statement for what to do when those values are caught.
Example Question #1 : Interface Declarations
Which of the following declares an interface to be used for anything that can implement the actions of a sink?
public interface Sink {
public void Turn(int faucetNum);
public void Drain();
public void Autoclean(int setting);
}
public abstract class Sink {
abstract public void Turn(int faucetNum);
public void Drain() {
// Drain code...
};
abstract public void Autoclean(int setting);
}
public abstract class Sink {
abstract public void Turn(int faucetNum);
abstract public void Drain();
abstract public void Autoclean(int setting);
}
public interface Sink {
public void Turn(int faucetNum) {
// Turn code... excerpted
}
public void Drain() {
// Drain code... excerpted
};
abstract public void Autoclean(int setting) {
// Auto-clean code... excerpted
}
}
public interface Sink {
public void Turn(int faucetNum);
public void Drain() {
// Drain code...
};
public void Autoclean(int setting);
}
public interface Sink {
public void Turn(int faucetNum);
public void Drain();
public void Autoclean(int setting);
}
First of all, the question does ask for an interface. This is a type of declaration in Java, so you should use that for your answer. This allows you to eliminate two of the incorrect options. Granting this, you then know that you need to look at the options that use the interface keyword. Recall that for interfaces, you provide no implementation whatsoever. Thus, the other two wrong answers are the ones that provide method bodies. This cannot be done for interfaces, which merely define what something should do but do not actually implement it.
Example Question #21 : Program Implementation
Design an interface Cat. The interface must include the functions meow, purr, clean, hungry, isPurring, isClean, isSleeping, and isHungry.
interface Cat {
}
class Cat {
public String meow();
public String purr();
public void clean();
public void hungry();
public boolean isPurring();
public boolean isClean();
public boolean isSleeping();
public boolean isHungry();
}
interface Cat {
public String meow();
public String purr();
public void clean();
public void hungry();
public void isPurring();
public void isClean();
public void isSleeping();
public void isHungry();
}
interface Cat {
public String meow();
public String purr();
public void clean();
public void hungry();
public boolean isPurring();
public boolean isClean();
public boolean isSleeping();
public boolean isHungry();
}
interface Cat {
public String meow();
public String purr();
public void clean();
public void hungry();
public boolean isPurring();
public boolean isClean();
public boolean isSleeping();
public boolean isHungry();
}
The correct answer defines the interface as described. One of the answer choices defines a class with all of the attributes of the interface. Classes and interfaces are different. Interfaces are stubs of methods and classes are typically implementations (this is not always true, look up abstract classes). Another answer choice defines just the stub of the interface. This one would have been correct if the prompt had just asked for the stub, but there were implementation questions involved. Finally, the third answer choice had isPurring(), isClean(), isSleeping(), and isHungry() as void methods. These methods are prefixed with an "is" and "is" typically defines boolean language. Boolean was the correct return method on those.
Example Question #173 : Computer Science
#include <iostream>
#include <string>
using namespace std;
int main() {
string name;
cout << "Tell me your name: "<<endl;
getline(cin, name);
sayHello();
return 0;
}
void sayHello(string nameToGreet){
cout << "Hello, " << nameToGreet << "!"<< endl;
}
Why would the above program fail?
name
is not the right type.
sayHello
doesn't exist at the point it is called.
There is no problem.
sayHello
should not be of type void
.
nameToGreet
was never defined.
sayHello
doesn't exist at the point it is called.
The compiler will read the program from top to bottom, and by the time it gets to the function call for sayHello
in main, it will have no clue what sayHello
is. Therefore an exception will be raised. To circumvent this issue, define sayHello
before main, OR provide a function prototype before main to make the compiler aware of the appropriate signature for the sayHello
function.
Example Question #174 : Computer Science
Which of the following is a method that takes a string and an integer as parameters, using the integer to increase each character value in the string before returning the new value? (For instance, a 2 applied to abc should become cde.)
public String myMethod(String s,int i) {
String r = "";
for(int j = 0; j < s.length(); j++) {
r += (char)(s.charAt(j) + i);
}
return r;
}
public String myMethod(String s,int i) {
String r = "";
for(int j = 0; j < s.length(); j++) {
r += (s.charAt(j) + i);
}
return r;
}
public void myMethod(String s,int i) {
String r = "";
for(int j = 0; j < s.length(); j++) {
r += (char)(s.charAt(j) + i);
}
}
None of the others
public int myMethod(String s,int i) {
String r = "";
for(int j = 0; j < s.length(); j++) {
r += (char)(s.charAt(j) + i);
}
return r.length();
}
public String myMethod(String s,int i) {
String r = "";
for(int j = 0; j < s.length(); j++) {
r += (char)(s.charAt(j) + i);
}
return r;
}
The first thing you need to look at is the definition of the function's return value and parameters. The only options that will pass are the ones that have:
public static String myMethod(String s,int i)
(This is based on the definition: Return a string, take a string and an integer.)
Now, in our code, the key line is:
r += (char)(s.charAt(j) + i);
The wrong version has:
r += (s.charAt(j) + i);
This does not work because s.charAt(j) + i is interpreted as a numeric value in this case. You will end up with a String that is comprised of numbers. However, if you cast it back to a char type, you will get the character that is defined by that particular numeric value. This is why the correct answer has:
r += (char)(s.charAt(j) + i);
Now, on the AP Computer Science A exam, you will need to use casts for double and int. However, the writers of the test do say that it is helpful to understand casting in general. Therefore, apply this problem's methodology to other programming problems where you would have to type cast double values into int values and vice-versa.
Example Question #2 : Method Declarations
1. class test
2. {
3. public:
4. test();
5. exams(int);
6. quiz(int);
7. private:
8. int grade;
9. }
Which line represents the constructor?
6
1
3
4
8
4
The constructor looks like a function declaration but always has the same name of the class.
Example Question #22 : Program Implementation
Fill in the blank.
A ______ function does not specify a return type, but may have any number of inputs.
void
double
empty
constructor
int
void
Remember, a void function does not need a return type. The void keyword tells the program not to expect a return statement. A void function does not necessarily mean that the inputs are void as well. A void function can still have any number of inputs of any type.
Example Question #23 : Program Implementation
Write the stub for a public method named writeToConsole that takes in a boolean b and a string s that returns an integer.
public writeToConsole(boolean b, string s)
public void writeToConsole(boolean b, string s)
public int writeToConsole(boolean b, string s)
public int writeToConsole()
public int writeToConsole(boolean b, string s)
Public methods are prefixed with the word public. The second word is the return type (i.e. int). The third is the name of the method and inside the parentheses goes the inputs to the method (i.e. boolean b and string s).
Example Question #1 : Method Declarations
True or False.
This code snippet defines a method that takes in two strings as input and will return a boolean as a result.
public void returnBoolean (String input1, String input2);
False
True
False
The prompt specifies that the return type of the method is a boolean. The return type of the method in the code snippet is void. Therefore, it will not return anything. The two input types however are correct.
Example Question #23 : Programming Constructs
CONSTRUCTORS
Consider the following Java code:
class Person
{
String firstName;
String lastName;
int age;
Person(String l, String f, int a)
{
firstName = f;
lastName = l;
age = a;
}
}
In main, how can I correctly create a person who is 21 years old, whose first name is Indiana, and whose last name is Jones?
Person person1 = new Person("Jones", "Indiana", "21");
Person person1 = new Person();
Person person1 = new Person("Jones", "Indiana", 21);
Person person1 = new Person("Indiana", "Jones", 21);
Person person1 = new Person("Jones", "Indiana", 21);
When we take a look at the constructor, first we notice that it has three parameters. Since there isn't any constructor overloading, this means that we can only create a person if we provide all of the arguments. So we can eliminate Person person1 = new Person() because that answer is not providing any arguments.
Now, we are supposed to be providing first two String arguments and then an integer. Therefore, we can eliminate the following two answers:
Person person1 = new Person("Jones", "Indiana", "21");
Person person1 = new Person(21, "Indiana", "Jones");
We can eliminate the first because the number 21 is being passed as a String instead of an int. It's being passed as a String because it has the double quotation. We can also eliminate the second because an integer is being passed as the first argument, instead of the third.
Lastly whenever a new object is created, the order of the arguments matter! Notice that the constructor is assigning the first parameter "l" to lastName. Therefore, our first argument should be "Jones". Next, the constructor takes the second parameter "f" and assigns it to the variable firstName. This means that our second argument should be "Indiana". Lastly, the constructor takes the third parameter "a" and assigns it to the integer variable age. This means that the last argument we should pass is the number 21 (without any quotes). This means that the correct answer is:
Person person1 = new Person("Jones", "Indiana", 21);
Certified Tutor
All Computer Science Resources
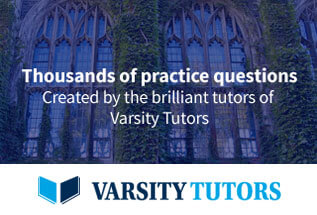