All Computer Science Resources
Example Questions
Example Question #1 : Console Output
What is the output of this program?
arr = ["hello", "my", "name", "is"]
for (int i = 0; i < arr.length - 1; i++) {
System.out.println(arr[i]);
}
System.out.println("Sandra");
hello my name is Sandra
hello my name is
hello
my
name
is
Sandra
Sandra
hello
my
name
is
Sandra
The words are printed on separate lines due to the System.out.println() call and then whatever is inside of the parentheses is printed on the next line. This call is different from System.out.print() which prints words on the same lines.
Therefore, the Sysytem.out.printIn gives("Sandra"):
hello
my
name
is
Sandra
Example Question #2 : Console Output
True or False.
The output of this code snippet will be "Hello, I'm hungry!"
public static void meHungry() {
String hungry = "hungry";
String iAm = "I'm";
String hello = "Hello";
String message = "";
if (hungry != null) {
message += hungry;
}
if (hello != null && iAm != null) {
message = hello + iAm + hungry;
}
System.out.println(message);
}
False
True
False
The message that is printed out is "Hello I'm hungry"
Notice there is no punctuation in the message. The code does not add punctuation to the message, but prints the words out in the same order as the phrase in the prompt. Be mindful of what's actually happening in the code.
Example Question #41 : Program Implementation
In Swift (iOS), give a description of what this method does.
func getDivisors(num: Int, divisor: Int) -> Bool {
var result: Bool = False
if (num % divisor == 0) {
result = True
}
println(result)
}
This function doesn't do anything.
This method returns true if the number is evenly divisible by the divisor. It returns false otherwise.
This function returns false if the number is evenly divisible by the divisor. It returns true otherwise.
This function returns true or false.
This method returns true if the number is evenly divisible by the divisor. It returns false otherwise.
The modulus function "%" determines the remainder of a division. If I have 1 % 2, the remainder is 1. If I have 2 % 2, the remainder is 0. Therefore, if the remainder is equal to 0, then the number is divisible by the function. Thus, the method returns true if the number is evenly divisble by the divisor and false otherwise.
Example Question #2 : Console Output
Does the code compile and if yes, what is the the output?
public class Test
{
public static void main ( String [] args )
{
int x = 2;
if (x = 2)
System.out.println("Good job.");
else
System.out.println("Bad job);
}
}
No, it doesn't compile.
Yes it compiles and it prints good job.
Yes it compiles and it prints bad job.
No, it doesn't compile.
It doesn't compile. It is supposed to be x == 2 within the if statement not x = 2. The if statement checks for booleans (only true or false) and cannot convert an integer into a boolean to meet that check.
Example Question #1 : Iterations
int var=0;
int array[4];
for (int j=0; j<=4;j++)
{
var=var+1;
}
What is the value of var?
10
5
6
8
4
5
The for loop simply sums up the numbers 0 through 3, which is 6.
First iteration through the loop, j=0:
var=0+1=1
Second iteration, j=1:
var=1+1=2
Third iteration, j=2:
var=2+1=3
Fourth iteration, j=3:
var=3+1=4
Fifth iteration, j=4:
var=4+1=5
Example Question #41 : Programming Constructs
public static boolean remove(int[] arr, int val) {
boolean found = false;
int i;
for(i = 0; i < arr.length && !found; i++) {
if(arr[i] == val) {
found = true;
}
}
// START
if(found) {
for(int j = i; j < arr.length;j++) {
arr[j - 1] = arr[j];
}
arr[arr.length - 1] = 0;
}
// END
return found;
}
What does the code between // START
and // END
do?
Delete the values in the array
None of the other options
Overwrite the array values with default values
Set the deleted index's value to 0
Shift the contents of the array
Shift the contents of the array
The easiest way to approach this question is to comment on this line by line. Notice that the condition only works if the given value was found. (This is the use of the boolean found
.) If the desired value has indeed been located, the code then goes through the array, picking up just past the location of the index. (At this point, i
is one past the index of the value to be deleted.) Then, it goes through the code and moves backward the value at each index. In effect, this shifts the content backward, giving the appearance of having performed a deletion. This is done on the line arr[j - 1] = arr[j];
. Finally, at the end, the final index value is set to a default value, namely 0. (This is done on the line arr[arr.length - 1] = 0;
.)
Example Question #1 : Iterations
#include <iostream>
using namespace std;
int main()
{
int i=0;
int sum =0;
for(i;i<4;i+=2)
{
sum=sum+i;
i--;
}
return 0;
}
What is the value of i and sum?
i=4, sum=6
i=3, sum=8
i=3,sum=6
i=4,sum=4
i=5,sum=7
i=3,sum=6
Let's take a look at the declaration of the foor loop. It will run as long at the integer "i" is less than four. It also increases by 2 after each iteration. i+=2 is equivalent to i=i+2.
Now let's look at the contents of the loop itself. The integer "sum" starts at 0 and is added to i at each iteration. After this we see that 1 number is subtracted from i after each iteration. Now after examining the structure of the loop, let's begin to calculate each iteration.
Iteration 1: i=0, sum=0, then i=-1.
Iteration 2: i=1, sum=1, then i=0.
Iteration3: i=2, sum=3, then i=1,
Iteration 4: i=3, sum =6, then i=3.
There is no iteration 5 because "i" can only be less than four to enter the loop, so the program ends there.
i=3, sum=6
Example Question #1 : Iterations
True or False.
This code snippet will iterate 5 times.
ArrayList<String> arrList = new ArrayList<String>();
arrList.add("string0");
arrList.add("string1");
arrList.add("string2");
arrList.add("string3");
arrList.add("string4");
for (int i = 0; i < arrList.size(); i++) {
System.out.println(arrList.get(i));
}
True
False
True
The ArrayList is populated with 5 strings. The for loop will iterate through the ArrayList from position 0 to 4 because once i gets to 5 the loop with exit. 0 to 4 is five iterations. So the answer is true.
Example Question #201 : Computer Science
Suppose you have the following code:
public static void main(String[] args) {
int a =2;
if (a%2==0)
System.out.println("Hello World");
else
System.out.println("Hi");
}
If the main method is called, what will be printed?
Hello World Hi
Hi
An error will be thrown
Hello World
Hi
Hello World
Hello World
"Hello World" will be printed, since the first condition is true: 2%2=0, or equivalently 2 is an even number. Once a condition in an if block is executed, the if block is exited. This means that any other elseif or else clauses will not be executed. If a%2==0 were False, then "Hi" would be printed. In no situation would it be possible for both "Hello World" and "Hi" to be printed. Additionally, no errors would be thrown since the syntax is correct and no runtime errors occur.
Example Question #42 : Program Implementation
Suppose you are given the following lines of code (and x is some initialized integer):
int k = arr.length;
for (int i = -1; i <k-2; i++)
{
if (arr[i+2] < x)
System.out.print("Hello");
}
What is the maximum number of times "Hello" print?
An ArrayIndexOutOfBoundsException will be thrown
k-1
k-2
0
k
k-1
The answer is k-1, where k is the length of the array.
This problem tests the students' understanding of loop itterations. The loop counter begins at -1, but the first element of arr to be accessed is at index=1. Likewise, the loop exits when i<k-2 is no longer true, and the final index of arr to be accessed is at index=k-3+2=k-1, which is the last element of arr. Therefore, we do not get an ArrayIndexOutOfBoundsException because the array is being accessed properly.
Since the (k-1)th element of arr is accessed (the last element) and the 0th element of arr is skipped (the first element to be accessed is at index=1, and arrays are 0 indexed), there are k-1 loop itterations. If all the values in arr do not exceed x, then "Hello" will printed k-1 times.
Certified Tutor
All Computer Science Resources
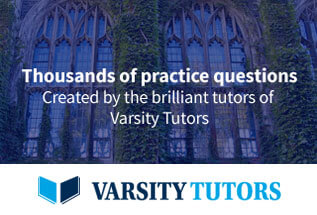