All Computer Science Resources
Example Questions
Example Question #22 : Object Oriented Program Design
Consider the code below:
private static class Philosopher {
private String name;
private String favoriteSubject;
public Philosopher(String n, String f) {
name = n;
favoriteSubject = f;
}
public String getName() {
return name;
}
public String getFavoriteSubject() {
return favoriteSubject;
}
public void speak() {
System.out.println("Hello, World! My name is "+name + ". My favorite subject is "+favoriteSubject);
}
}
private static class Nominalist extends Philosopher {
boolean franciscan;
public Nominalist(String n,boolean frank) {
super(n,"logic");
franciscan = frank;
}
public void speak() {
super.speak();
if(franciscan) {
System.out.println("I am a Franciscan");
} else {
System.out.println("I am not a Franciscan");
}
}
public String whoMightHaveTaughtMe() {
if(franciscan) {
return "Perhaps William of Ockham?....";
} else {
return "Perhaps it was Durandus of St. Pourçain — scandalous, a Dominican nominalist!";
}
}
}
If you wished to make a toString() method for both Philosopher and Nominalist, using the same output as in each class' current speak() methods, what would be the best way to organize your code?
I. Have toString() invoke both classes' speak() methods.
II. Have the speak() method invoke the new toString() method.
III. Create a new subclass and write the toString() method in that.
III
I
I and III
II
II
The toString() method must return a string value. Therefore, it makes the most sense that you would have this method generate the output that you currently generate in speak(). Then, you could have speak() invoke the toString() method to output the same string data.
Example Question #302 : Computer Science
Given a class Thing and the code:
Thing thingOne, thingTwo;
What function call is the following equivalent to?
thingTwo = thingOne;
None of the above
operator=(thingTwo, thingOne);
thingTwo.operator = (thingOne);
thingOne uses the copy constructor of thingTwo
ostream& Thing::operator=(const Thing& rhs);
thingTwo.operator = (thingOne);
What's given to us is that thingOne and thingTwo have already been created, this is a vital piece of information. Let's go through each of the choices
operator=(thingTwo, thingOne);
This line of code makes no sense and is syntactically wrong.
ostream& Thing::operator=(const Thing& rhs);
This line of code says to access the "operator=" method from the "Thing" class and put it onto the ostream, this has nothing to do with assigning thingOne to thingTwo.
When we come across the choice of the copy constructor, we have to keep in mind that the copy constructor is only used when an object use to to create another one, for example we have a given class called Foo:
Foo foo;
Foo foobar = foo;
In this case, we are using foo to create foobar.
The only choice that works is:
thingTwo.operator=(thingOne);
This line of code means that the thingOne object is being assigned to thingTwo.
Example Question #1 : Program Design
Consider the history of the following popular programming languages:
PHP
Java
Objective-C
Python
Which of the following is the closest ancestor shared by ALL of these languages?
Ruby
C
Smalltalk
Ada
Lisp
C
All of these languages are C-based languages.
- Ruby was invented in 1995, the same year as PHP, so it could not have influenced earlier languages like Objective-C and Python.
- Lisp did influence at least one language, Python, but it did not influence any others.
- Ada directly influenced Java, and because it influenced C, it can be argued that it is an ancestor of these other languages; however, because of this, Ada is not the CLOSEST ancestor.
- Smalltalk influenced Objective-C, but no other languages on this list.
A clue was the answer "Objective-C," which is a strict superset of C that adds Object Orientation.
Example Question #304 : Computer Science
What is the value of the string kitchen after the following code is run?
- class home
- {
- public:
- home(string);
- void searchhome();
- int buyhome();
- private:
- string kitchen();
- };
- home::home(string c)
- {
- kitchen=c;
- }
- int main()
- {
- str=’big’;
- home(str);
- }
str
'small'
'big'
void
c
'big'
The constructor here in line 4 of the class definition is where it gets tricky. In the initialization of the constructor, we note that the input is a string.
Going down to line 10, to where the constructor function is defined, we see that a constructor with an input of c, which is defined as a string, will set the value of kitchen to c.
Finally, going down to our main code, we see that the value of the constructor in main is 'big', defined in str.
So kitchen='big'.
Example Question #1 : Program Design
What is the difference between declaring a function as void and declaring it as int?
An int function needs to declare an integer variable inside the function and a void function doesn't.
A void function has no input and an int function has at least one integer input.
A void function can have any return type whereas an int function can only have one return type.
There is no difference.
A void declaration does not expect an output to that function, whereas an int function declarations expects an intger value as the output to the function.
A void declaration does not expect an output to that function, whereas an int function declarations expects an intger value as the output to the function.
Function delcarations are very significant, as you must define what the return type of the function is. A void function doesn't need a return type, whereas the int function must have an integer value as its return type.
Example Question #2 : Program Design
What's wrong with the following code?
void printsometext()
{
cout<<"printing text\n";
for(int i=0;i<3;i=i+1)
{
cout<<i<<"\n"
}
return 2;
}
A void function cannot return an output.
The character \n does not exist.
The loop is formatted incorrectly.
Cout cannot output a number.
You need semicolons after the function declaration.
A void function cannot return an output.
It is very important when creating a function to select the return type. If you want your function to return an integer, you would place "int" before your function to signify that the function expects an integer output. In this case, the function is declared as void, meaning there is no output. However, the code indicated a return value of 2, which conflicts with the void keyword. To fix this problem, either remove the return statement or change void to int.
Example Question #5 : Program Design
CHOOSING LOOPS
Imagine that I want to write a code for a bank that asks a user whether he/she wants to make a withdrawal, make a deposit, or quit. What type of loop would be best to use in order to make sure the user is able to do as many transactions as they want until they press quit to end the program?
A do-while loop.
A for loop.
An infinite loop.
A while loop.
A do-while loop.
In our problem, the program has to run AT LEAST ONCE in order to present all of the options to the user. Because of this reason, the best option is a do-while loop because the statements within that loop execute at least one time and the condition to get out of the loop is at the end. In this case, the exit condition would be if the user presses quit (after the options have been shown at least once).
A while loop might work in this case; however it is not necessarily the smartest option. This is because the condition is tested before anything is executed within the loop. This means that whatever is inside the while loop does not necessarily have to be executed at all.
A for loop is also not a good option for this problem because a for loop is executed a set amount of times. In this case, we don't know if the user is going to want to do 1 transaction or 10. Therefore, a for loop is not the best choice.
An infinite loop is also not what we want because it runs forever. In our case, we want the code to stop prompting the user and end after "quit."
Example Question #311 : Computer Science
USING POINTERS
Study the following pseudocode.
int * var1;
int foo = 63;
var1 = &foo;
var2 = *var1;
What are the values of var1 and var2?
var1 is assigned a value of 63
var2 is assigned a value off 63
var1 is assigned the memory address value of foo
var2 is assigned the memory address value of var1
var1 is assigned a value of 63
var2 is assigned the memory address value of var1
var1 is assigned the memory address value of foo.
var2 is assigned a value of 63
var1 is assigned the memory address value of foo.
var2 is assigned a value of 63
Pointers store the address of another variable. Pointers are declared by naming the type, then an asterisk, followed by the name of the variable. In our example, var1 was declared a pointer that points to an integer:
int * var1;
Next in the code, we see that an integer variable named "foo" is created and is assigned a value of 63.
The address-of operator (&) is then used to get the address of a variable. Now lets take a look at the next line of the code:
var1 = &foo;
Here the ampersand is used to get the address of foo within memory. This means that var1 contains the address value of where foo is stored in memory.
Next in the code, we have the following statement:
var2 = *var1;
Here the dereference operator (*) is being used. This operator is used whenever we want to get the value (not the address) of the variable that a pointer is pointing to. In this case, var1 is storing the address of foo. However, by using the dereference operator we can get the actual value of the variable whose address is being stored in var1. In this case, dereferencing var1, we get the actual value of foo which is 63. Therefore, var2 = 63.
Example Question #1 : Arrays
TWO DIMENSIONAL ARRAYS
Given the following initialized array:
int fourth;
int[][] myArray = { {1, 2, 3},
{4, 5, 6},
{7, 8, 9} };
Using myArray, how can I store in variable "fourth", the number 4?
fourth = myArray[2][1];
fourth = myArray[1][1];
fourth = myArray[0][1];
fourth = myArray[1][0];
fourth = myArray[1][0];
When a two dimensional array is created and initialized, the way to access the items inside the matrix is by calling the array with the row and column (i.e. myArray[ROW][COLUMN]). Keeping in mind that arrays start at 0, the number four would be in row 1, column 0. Therefore to save that number into the variable "fourth" we'll do the following:
fourth = myArray[1][0];
*Note: myArray[1][0] is not the same as myArray[0][1].
myArray[1][0] =4 because it is the item located at row=1 and column = 0.
myArray[0][1] =12 because it is the item located at row=0 and column = 1.
Example Question #9 : Program Design
What would be the best data structure for a library? The data is in the form of a title and a number of copies of the title.
Array
Hash map
Static values
ArrayList
Hash map
Hash map - key being title and value being the number of copies
Hash maps are a collection of (key, value) pairs.
Hash maps have O(1) access, so this would be the quickest and best way to store the data.
All Computer Science Resources
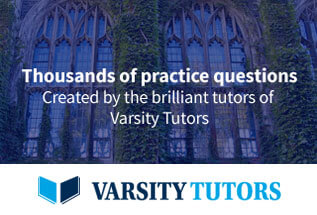