All Computer Science Resources
Example Questions
Example Question #21 : Standard Data Structures
#include <iostream>
using namespace std;
bool bigger(int x, int y)
{
if (x>y)
return true;
else
return false;
}
int main()
{
bool test;
test!=bigger(10,7);
cout<<test;
}
What will be the value of test after the previous code is run?
0
false
10
true
7
0
The function "bigger" defined before main returns a value of type bool and takes in two integer inputs. It compares the first value to the second. If it it greater, it returns true. Otherwise, it returns false. In main, a new variable test is called, and it is of type bool as well. This automatically eliminates 3 out of the 5 possibilities.
"test" is defined as the opposite of the outcome of the bigger function with the two inputs are 10 and 7. 10 is bigger than 7, so the function returns true. Since test is the opposite of that, it is false.
Example Question #2 : Boolean
Convert the decimal number 67 to binary.
1000010
1000101
1100011
1000011
1001010
1000011
In a regular number system, also known as decimal, the position furtherest to the right is , then one over to the left would be
, then
. These are the ones, tens and hundreds place. Binary is a base 2 number system. Therefore, the digit to the furthest right is
, then to the left
, then
, and so on.
Explanation
1000011 The bolded number has a value of 1
1000011 The bolded number has a value of 2
1000011 The bolded number has a value of 64
The positions that are marked true (as 1) in the binary number 1000011 corresponds to the numbers 64, 2, and 1 which added up equals 67
Step By Step
- To convert the number 67 to binary, first find the digit place number that has the largest number possible that is less than or equal to 67. In this case it would be the position holding
.
- The number for 64 is 1000000
- To get 67, we need to add 3
- Again, find the digit place number that has the largest number possible less than or equal to 3. In this case, it would be
- The number for 66 (64 + 2) is 1000010
- To get from 66 to 67, repeat the steps from before.
- The number for 67 (66 + 1) is 1000011
Example Question #21 : Standard Data Structures
In Swift (iOS), define an unwrapped boolean.
variable: Boolean
var variable: Bool
variable: Bool
var variable
var variable: Bool
In Swift, every variable must be declared with var before the name of the variable. This automatically knocks out two of the answers. After we eliminate those two, we see var variable and var variable: Bool. var variable would be a correct answer if the prompt had not asked for an unwrapped boolean. var variable: Bool performs the unwrapping by declaring the variable to be a boolean specifically.
Example Question #22 : Standard Data Structures
How do we set a method to return a Boolean in Swift(iOS)?
func method() -> Bool {}
func method -> Bool {}
func method() -> Bool {}
method() -> Bool {}
method() -> Bool {}
func method() -> Bool {}
In Swift, all methods must first say what they are. They are functions, so they are prefixed with func. Next, methods must have a name. In this case, we named it method. All methods need to specify parameters, even if there are no parameters. So, method() i.e. no parameters. Finally, we wanted to return a boolean. So we set the return type using -> Bool.
Example Question #22 : Standard Data Structures
How do we set a method to return a Boolean in Swift(iOS)?
func method() -> Bool {}
func method -> Bool {}
func method() -> Bool {}
method() -> Bool {}
method() -> Bool {}
func method() -> Bool {}
In Swift, all methods must first say what they are. They are functions, so they are prefixed with func. Next, methods must have a name. In this case, we named it method. All methods need to specify parameters, even if there are no parameters. So, method() i.e. no parameters. Finally, we wanted to return a boolean. So we set the return type using -> Bool.
Example Question #71 : Computer Science
What line number makes the following Python Code not execute? (1:, 2:, 3:,... represents line numbers)
1: x=[]
2: y=[]
3: z=[]
4: for i in range(10):
5: x.append(i)
6: y.append(x[i]+2*x[i])
7: z.append(x[i]+y[i]**2)
8: print(z)
9: string="The fourth element of z is "+z[3]
10: print(string)
Line 8
Line 5
Line 7
Line 6
Line 9
Line 9
When the code is executed it will produce a type error with the variable string in line 9. The variable string is trying to concatenate a string and an integer from the z array. To make the code executable, we would need to make the integer from the z array a string. We would do this by simply wrapping it in the method str(), which converts it into a string. After making the change, the following code will execute correctly.
1: x=[]
2: y=[]
3: z=[]
4: for i in range(10):
5: x.append(i)
6: y.append(x[i]+2*x[i])
7: z.append(x[i]+y[i]**2)
8: print(z)
9: string="The fourth element of z is"+str(z[3])
10: print(string)
Example Question #2 : Double
Consider the following code:
double a = 4.5, d = 4;
int b = 10,c=5;
double e = a / b + c / d - d % c;
What is the value of e
at the end of this code's execution?
There is a type-related error in the code.
The easiest way to work through this code is to comment on its parts. See the comments below in bold.
double a = 4.5, d = 4;
int b = 10,c=5;
/*
a / b: This is a division that will maintain the decimal portion (since it has a double involved in it). 4.5 / 10 will evaluate to 0.45.
c / d: Since this has both an int and a double, it will evaluate to a double. That means that it will maintain its decimal portion as well. It is: 5 / 4 or 1.25.
d % c: Since d is 4, this kind of remainder division works just like having two integers for the modulus. 4 % 5 is just 4. (It is 0 remainder 4.)
Thus, the expression is: 0.45 + 1.25 - 4, which is -2.3.
*/
double e = a / b + c / d - d % c;
Example Question #3 : Double
What is the size of the float
data type in Java?
4 bytes (32 bits)
1 byte (8 bits)
2 bytes (16 bits)
6 bytes (48 bits)
8 bytes (64 bits)
4 bytes (32 bits)
A float
is represented by 4 bytes (32 bits). It's comprised of 1 sign bit, 8 exponent bits, and 23 mantissa bits. An example of a floating point number in binary would be 11111111111111111111111111111111
. The breakdown would be [1](sign bit) [11111111](exponent bits) [11111111111111111111111](mantissa bits)
. All in all, that equals 32 bits.
In Java, the byte
is the only 8 bit data type. The short
and char
are 16 bits in size. There are no 48 bit data types, as everything is in a power of 2. The double
and long
are 64 bits in size.
Because floats
have half the number of bits as doubles
, it isn't as precise, so shouldn't be used when lots of precision is needed. It's better suited for when there are memory size concerns.
Example Question #21 : Standard Data Structures
Breanna wants to keep track of how many grapes she eats in a day over the course of a week.
Her brother Nathan, however, wants to keep track of the average amount of grapes he eats in a day over the course of a week.
What type of variable would best be fit for what Breanna wants to keep track of? What about Nathan?
Breanna should use double
Nathan should use int
Breanna should use int
Nathan should use int
Breanna should use double
Nathan should use double
Breanna should use int
Nathan should use double
Breanna should use int
Nathan should use double
Breanna should use int because she would be counting whole numbers.
Nathan however, should use double because he will be adding up how many grapes he eats and divide by the number of days in the week.
Example Question #23 : Standard Data Structures
How do we declare a method to return a Double in Swift (iOS)?
func method() -> Double {}
method() -> Double {}
func method -> Double {}
func -> Double {}
func method() -> Double {}
In Swift, all methods must first say what they are. They are functions, so they are prefixed with func. Next, methods must have a name. In this case, we named it method. All methods need to specify parameters, even if there are no parameters. So, method() i.e. no parameters. Finally, we wanted to return a Double. So we set the return type using -> Double.
Certified Tutor
Certified Tutor
All Computer Science Resources
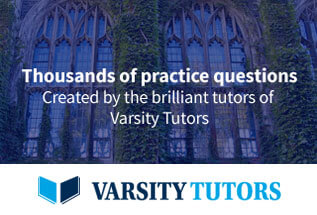