All Computer Science Resources
Example Questions
Example Question #1 : Common Data Structures
Consider the following code:
int[] vals = {5,4,2};
String s = "Hervaeus";
String s2 = "";
for(int i = 0; i < s.length(); i++) {
for(int j = 0; j < vals[i % vals.length]; j++) {
s2 += s.charAt(i);
}
}
System.out.println(s2);
What is the output for the code above?
HHHHHeeeerrvaeus
The code will note execute.
HHHHHeeeeerrrrrrvvvvvaaaaaeeeeeuuuuusssss
Hervaeeeeeuuuuss
HHHHHeeeerrvvvvvaaaaeeuuuuussss
HHHHHeeeerrvvvvvaaaaeeuuuuussss
The main thing to look at for this question is the main loop for the code. This loop first goes through each of the characters in the String s:
int i = 0; i < s.length(); i++
Next, notice the condition on the inner loop:
vals[i % vals.length]
The modulus on i will yield values that are between 0 and 2 (given the length of vals). This means that you will loop in the inner loop the following sequence of times:
5,4,2,5,4,2,5,4
This will replicate the given letter at the index (i) in the initial string, using 5,4,2,5, etc as the replication count. Thus, you will replicate "H" 5 times, "e" 4, etc. This gives you an output:
HHHHHeeeerrvvvvvaaaaeeuuuuussss
Example Question #1 : Common Data Structures
Consider the following code:
char[] values = {'I',' ','l','o','v','e',' ','y','o','u','!','!'};
String s = "";
for(int i = 0; i < values.length / 2; i++) {
char temp = values[i];
values[i] = values[values.length - i-1];
values[values.length - i-1] = temp;
}
for(int i = 0; i < values.length; i++) {
s += values[i];
}
System.out.println(s);
What is the output for the code above?
!uyeolI!o vl
!!uoy evol I
None of the others
!!you love I
!you I love!
!!uoy evol I
It is easiest to think of the values array as a String: "I love you!!".
Now, the loop is going to run for or
times. Notice what it does on each iteration. It swaps the values at i and values.length - i -1. Thus, it will do the following swaps:
0 and 11
1 and 10
etc...
This sequence of swaps will eventually reverse the array. Thus, your output is:
!!uoy evol I
Example Question #2 : Common Data Structures
Which of the following blocks of code makes every other character in the string s to be upper case, starting with the second character?
String s = "This is a great string!";
String s2 = "";
for(int i = 0; i < s.length(); i++) {
char c = Character.toLowerCase(s.charAt(i));
if(i % 2 == 1) {
c = Character.toUpperCase(c);
}
s2+=c;
}
String s = "This is a great string!";
String s2 = "";
for(int i = 0; i < s.length(); i++) {
char c = Character.toLowerCase(s.charAt(i));
if(i % 2 == 3) {
c = Character.toUpperCase(c);
}
s2+=c;
}
String s = "This is a great string!";
String s2 = "";
for(int i = 0; i < s.length(); i++) {
char c = Character.toLowerCase(s.charAt(i));
if(i % 2 == 0) {
c = Character.toUpperCase(c);
}
s2+=c;
}
String s = "This is a great string!";
String s2 = "";
for(int i = 0; i < s.length(); i++) {
char c = Character.toLowerCase(s.charAt(i));
s2+=c;
if(i % 2 == 0) {
c = Character.toUpperCase(c);
}
}
String s = "This is a great string!";
String s2 = "";
for(int i = 0; i < s.length(); i++) {
char c = s.charAt(i);
if(i % 2 == 1) {
c = Character.toUpperCase(c);
}
s2+=c;
}
String s = "This is a great string!";
String s2 = "";
for(int i = 0; i < s.length(); i++) {
char c = Character.toLowerCase(s.charAt(i));
if(i % 2 == 1) {
c = Character.toUpperCase(c);
}
s2+=c;
}
Given that strings cannot be internally modified, you will have to store your result in a new string, namely s2. Now, for each character in s, you will have to make that charater lower case to begin with:
char c = Character.toLowerCase(s.charAt(i));
Next, for the odd values of i, you will need to make your value to be upper case. The modulus operator is great for this! You can use % 2 to find the odd values. When the remainder of a division by 2 is equal to 1, you know you have an odd value. Hence, you have the condition:
if(i % 2 == 1) {...
Then, once you appropriately capitalize, you can place your character on s2.
Example Question #1 : Common Data Structures
Which of the following blocks of code converts an array of characters into a string?
private static void string() {
char[] vals = {'A','t', ' ', '6',' ','a','m','!'};
String s = "";
for(int i = 0; i < vals.length; i++) {
vals[i] = s;
}
}
private static void string() {
char[] vals = {'A','t', ' ', '6',' ','a','m','!'};
String s = "";
for(int i = 0; i < vals.length; i++) {
s += vals[i];
}
}
private static void string() {
char[] vals = {'A','t', ' ', '6',' ','a','m','!'};
String s = "";
for(int i = 0; i < vals.length; i++) {
s = vals[i];
}
}
private static void string() {
String s = "At 6 am!";
char[] vals = new char[s.length()];
for(int i = 0; i < s.length(); i++) {
vals[i] = s.charAt(i);
}
}
private static void string() {
char[] vals = {'A','t', ' ', '6',' ','a','m','!'};
String s;
for(int i = 0; i < vals.length; i++) {
s += vals[i];
}
}
private static void string() {
char[] vals = {'A','t', ' ', '6',' ','a','m','!'};
String s = "";
for(int i = 0; i < vals.length; i++) {
s += vals[i];
}
}
The easiest way to consider this is by commenting on the correct answer. You must begin by defining the character array:
char[] vals = {'A','t', ' ', '6',' ','a','m','!'};
Next, you must initialize the string value s to be an empty string. This is critical. Otherwise, you can't build your string!
String s = "";
Next, you have the loop. This goes through the characters and concatenates the values to the variable s. The operation to concatenate the characters is the "+=". This will give you the string value of the array of characters.
Example Question #126 : Computer Science
See code below:
String[] books = {
"De Secundis Intentionibus",
"Leviathan",
"Averrois Commentaria Magna in Aristotelem De celo et mundo",
"Logica Docens for Idiots",
"Logica Utens for Tuba Players"
};
String userInput;
// In code excised from here, a person inputs the value "Logica Utens for Tuba Players" ...
for(int i = 0; i < books.length; i++) {
if(books[i] == userInput) {
System.out.println("This is a wonderful book!!");
}
}
What is the error in the code above?
The string comparison.
The use of the [] operator on the array.
It overruns an array.
The way the array is declared.
The use of if on an array element.
The string comparison.
The only major issue with this code is the use of the == operator to compare the two strings. Since the user has input this value, will not have an equality on this test. You must use the method .equals in order to check whether two strings are equal. The code should be:
if(books[i].equals(userInput)){
...
(There are some cases in which == will work for string comparison, namely when literals are involved. However, you should avoid relying on this and always use .equals().)
Example Question #62 : Standard Data Structures
String[] books = {
"Logica sive Ars Rationalis",
"Kritik der reinen Vernunft",
"Cursus Philosophicus Thomisticus",
"Happy words for happy people",
"Insane words for an insane world"
};
String str = "Kittens in a cart";
ArrayList<String> vals = new ArrayList<String>();
for(int i = 0; i < books.length; i++) {
if(books[i].compareTo(str) > 0) {
vals.add(books[i]);
}
}
System.out.println(vals);
What is the output for this method?
[Logica sive Ars Rationalis, Kritik der reinen Vernunft]
[Kritik der reinen Vernunft, Logica sive Ars Rationalis]
[Cursus Philosophicus Thomisticus, Happy words for happy people, Insane words for an insane world]
[]
[Happy words for happy people, Insane words for an insane world]
[Logica sive Ars Rationalis, Kritik der reinen Vernunft]
The compareTo method for strings compares to string objects and returns:
- 0 when they are equal
- Something positive when the given string is alphabetically (really, lexicographically) later than the argument to the method.
- Something negative when the given string is alphabetically (really, lexicographically) before the argument to the method.
Our strings could be put in this order:
"Cursus Philosophicus Thomisticus"
"Happy words for happy people"
"Insane words for an insane world"
"Kritik der reinen Vernunft"
"Logica sive Ars Rationalis"
For each of these, we are asking, "Is it later in order than 'Kittens in a cart'?" This is true for "Kritik der reinen Vernunft" and "Logica sive Ars Rationalis". Thus, our output is:
[Logica sive Ars Rationalis, Kritik der reinen Vernunft]
Notice the order—this is due to the order in the original array.
Example Question #1 : Strings
String greet = "Hello ";
String sub;
int len = greet.length();
sub = greet.substring(0, (len/2));
System.out.println (sub);
What is printed?
Error
Hello
He
Hel
Hell
Hel
The length of greet is 6 characters including the space at the end.
greet.substring(0, (len/2)) is equal to greet.substring(0, 3)
The substring of greet from the zeroth position to second position, not to the third position.
All Computer Science Resources
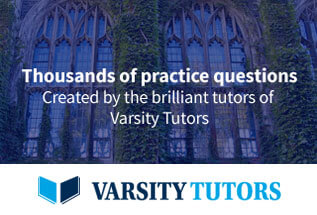