All Computer Science Resources
Example Questions
Example Question #2 : Debugging
Consider the following code:
Object[] objects = new Object[20];
for(int i = 0; i < objects.length; i++) {
switch(i % 4) {
case 0:
objects[i] = new Integer(i + 3);
break;
case 1:
objects[i] = "This val: " + i;
break;
case 2:
objects[i] = new Double(i * 4.4);
break;
case 3:
objects[i] = "That val: " + (i*12);
break;
}
}
String s = (String)objects[8];
System.out.println(s);
What is the error in the code above?
You cannot assign various types to the array in that manner.
There are no errors.
There will be a ClassCastException thrown.
There is an array overrun.
There will be a NullPointerException.
There will be a ClassCastException thrown.
In order to understand the error in this code, you must understand what the loop is doing. It is assigning variable types to the array of Object
objects based upon the remainder of dividing the loop control variable i
by 4. You thus get a repeating pattern:
Integer, String, Double, String, Integer, String, Double, String, . . .
Now, index 8 will be an Integer (for it has a remainder of 0). This means that when you do the type cast to a String, you will receive a TypeCastException for the line reading:
String s = (String)objects[8];
Example Question #2 : Run Time Errors
public static int[][] doWork(int[][] a, int[][] b) {
int[][] ret = new int[a.length][a[0].length];
for(int i = 0; i < a.length; i++) {
for(int j = 0; j < a[i].length; j++) {
ret[i][j] = a[i][j] + b[i][j];
}
}
return ret;
}
In the code above, what is the potential error that will not be caught on the following line?
ret[i][j] = a[i][j] + b[i][j];
The array could go out of bounds
The code is fine as it is written
The arrays may contain null values
The array a
may be set to null
The arrays may not be initialized
The array could go out of bounds
At this point of the code, it is not possible for a
or b
to be null. Furthermore, these arrays cannot contain null values, for they are made up of primitive types (int
types). These cannot be set to null, as they are not objects. The potential error here is a little bit abstruse, but it is important to note. There is one possible error, namely that the 2D arrays are "ragged"; however we don't need to worry about this for the exam. Still, it is also possible that the array b
(presuming that it is not null) is not the same size as the array a
. Since we are using a
to set our loop counts, this could potentially mean that we overrun the array b
by using index values that are too large. (Note, it is also possible that b
could be null, causing an error; however, there are no answer choices for that possibility.)
All Computer Science Resources
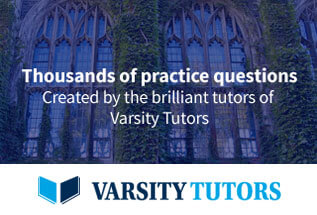