All Computer Science Resources
Example Questions
Example Question #1 : Object Oriented Program Design
The letters above the code samples are for the explanation.
Design a class in the programming language Swift (iOS) to best solve this problem:
I have a dog company. My company takes in dogs for boarding on the weekdays, weekends, and during holidays. I want my website to display when we're open, how many spots we have left, and the price for boarding.
(C)
class DogBoarding {
internal var open: Bool
internal var spotsLeft: Int
internal var price: Double
init (open: Bool, spotsLeft: Int, price: Double) {
self.open = open
self.spotsLeft = spotsLeft
self.price = price
}
internal func getPrice() {
return self.price
}
internal func getSpotsLeft() {
return self.spotsLeft
}
internal func isOpen() {
return self.open
}
}
(D)
class DogBoarding {
init () {
}
internal func getPrice() -> Double {
return 0.0
}
internal func getSpotsLeft() -> Int {
return 0
}
internal func isOpen() -> Bool {
return false
}
}
(B)
class DogBoarding {
private var open: Bool
private var spotsLeft: Int
private var price: Double
init (open: Bool, spotsLeft: Int, price: Double) {
self.open = open
self.spotsLeft = spotsLeft
self.price = price
}
private func getPrice() -> Double {
return self.price
}
private func getSpotsLeft() -> Int {
return self.spotsLeft
}
private func isOpen() -> Bool {
return self.open
}
}
(A)
class DogBoarding {
internal var open: Bool
internal var spotsLeft: Int
internal var price: Double
init (open: Bool, spotsLeft: Int, price: Double) {
self.open = open
self.spotsLeft = spotsLeft
self.price = price
}
internal func getPrice() -> Double {
return self.price
}
internal func getSpotsLeft() -> Int {
return self.spotsLeft
}
internal func isOpen() -> Bool {
return self.open
}
}
(A)
class DogBoarding {
internal var open: Bool
internal var spotsLeft: Int
internal var price: Double
init (open: Bool, spotsLeft: Int, price: Double) {
self.open = open
self.spotsLeft = spotsLeft
self.price = price
}
internal func getPrice() -> Double {
return self.price
}
internal func getSpotsLeft() -> Int {
return self.spotsLeft
}
internal func isOpen() -> Bool {
return self.open
}
}
Coding sample (A) is the correct answer. This is due to the completeness of the class. The class has getters for open, spotsLeft, and price which is what the user in the prompt asks for to be able to display them on a website.
Coding sample (B) is incorrect because everything is set to private. If the getter functions are private, then the user cannot use them for the website.
Coding sample (C) is incorrect because there are no return types on the functions. In Swift, the functions must have return types.
Coding sample (D) is incorrect because it is just the shell of a class and does not actual perform any functions.
Certified Tutor
All Computer Science Resources
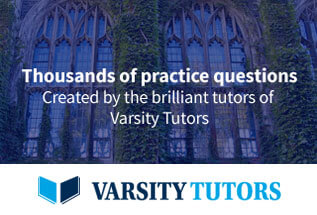