All Computer Science Resources
Example Questions
Example Question #1 : Object Oriented Program Design
Which of the following lines represents a data member?
1. class animal
2. {
3. public:
4. animal();
5. void fetch();
6. private:
7. char bone;
8. }
Line 3
Line 5
Line 7
Line 6
Line 1
Line 7
Data members are variables created in a class, and are typically found under the private: label, as data members are typically kept local to that class. It can be distinguished from member functions because of its lack of parenthesis().
Example Question #2 : Designing Classes
Which of the following operations allow you to define the function func1() outside of the class definition?
1. class school
2. {
3. public:
4. school();
5. void func1();
6. private:
7. char class;
8. }
->
=
,
::
;
::
The function template for func1() has been defined in the class definition, however the implementation of the function has not. In order to specify what the function does, you must say
classname::functionname()
{
}
Or, in our case,
school::function1()
{
//function definition goes in here
}
Example Question #2 : Object Oriented Program Design
Design a shell for a class named Dog that has 3 attributes:
- Does the dog have a tail?
- Is the dog purebred?
- A list of strings of definitive features for the dog
class Dog {
}
class Dog {
boolean purebred;
List features;
}
class Dog {
boolean tail;
boolean purebred;
}
class Dog {
boolean tail;
boolean purebred;
List features;
}
class Dog {
boolean tail;
boolean purebred;
List features;
}
In the prompt, any yes or no questions should be addressed as booleans (i.e. tail, purebred). Any lists should have the type and name of the list. This was just a shell, so there should be no declarations of variables.
Example Question #4 : Class Design
Design a class, SuperHero, that will be used for an existing application. The class should extend the parent class Man. The class should define clothes, skin color, hair color, eye color, good or evil, whether or not powers are had, and a list of personality traits. The class Man supports an implementation of the method doGood. Here is the method stub for doGood:
public String doGood(Boolean good);
Your class will need to support an implementation of doGood.
Choose the best answer.
class SuperHero extends Man {
List clothes;
String skinColor;
String hairColor;
String eyeColor;
Boolean good;
Boolean powers;
List personalityTraits;
public String doGood(Boolean good) {
String result = "No, do bad!";
if (good == true) {
result = "Yes, do good!";
}
return result;
}
}
class SuperHero {
List clothes;
String skinColor;
String hairColor;
String eyeColor;
Boolean good;
Boolean powers;
List personalityTraits;
public String doGood(Boolean good) {
String result = "No, do bad!";
if (good == true) {
result = "Yes, do good!";
}
return result;
}
}
class SuperHero extends Man {
List clothes;
String skinColor;
String hairColor;
String eyeColor;
public String doGood(Boolean good) {
String result = "No, do bad!";
if (good == true) {
result = "Yes, do good!";
}
return result;
}
}
class SuperHero extends Man {
List clothes;
String skinColor;
String hairColor;
String eyeColor;
Boolean good;
Boolean powers;
List personalityTraits;
public String doGood(Boolean good) {
}
}
class SuperHero extends Man {
List clothes;
String skinColor;
String hairColor;
String eyeColor;
Boolean good;
Boolean powers;
List personalityTraits;
public String doGood(Boolean good) {
String result = "No, do bad!";
if (good == true) {
result = "Yes, do good!";
}
return result;
}
}
This is the correct answer because the class extends the super class Man. It also lists all of the attributes defined in the prompt. In addition to listing all of the attributes, the class also defines an implementation of the doGood method which the prompt states is defined by the parent. One of the answer choices simply defined the stub for the method. The prompt clearly states to support an implementation of the method.
Example Question #3 : Object Oriented Program Design
The letters above the code samples are for the explanation.
Design a class in the programming language Swift (iOS) to best solve this problem:
I have a dog company. My company takes in dogs for boarding on the weekdays, weekends, and during holidays. I want my website to display when we're open, how many spots we have left, and the price for boarding.
(A)
class DogBoarding {
internal var open: Bool
internal var spotsLeft: Int
internal var price: Double
init (open: Bool, spotsLeft: Int, price: Double) {
self.open = open
self.spotsLeft = spotsLeft
self.price = price
}
internal func getPrice() -> Double {
return self.price
}
internal func getSpotsLeft() -> Int {
return self.spotsLeft
}
internal func isOpen() -> Bool {
return self.open
}
}
(C)
class DogBoarding {
internal var open: Bool
internal var spotsLeft: Int
internal var price: Double
init (open: Bool, spotsLeft: Int, price: Double) {
self.open = open
self.spotsLeft = spotsLeft
self.price = price
}
internal func getPrice() {
return self.price
}
internal func getSpotsLeft() {
return self.spotsLeft
}
internal func isOpen() {
return self.open
}
}
(D)
class DogBoarding {
init () {
}
internal func getPrice() -> Double {
return 0.0
}
internal func getSpotsLeft() -> Int {
return 0
}
internal func isOpen() -> Bool {
return false
}
}
(B)
class DogBoarding {
private var open: Bool
private var spotsLeft: Int
private var price: Double
init (open: Bool, spotsLeft: Int, price: Double) {
self.open = open
self.spotsLeft = spotsLeft
self.price = price
}
private func getPrice() -> Double {
return self.price
}
private func getSpotsLeft() -> Int {
return self.spotsLeft
}
private func isOpen() -> Bool {
return self.open
}
}
(A)
class DogBoarding {
internal var open: Bool
internal var spotsLeft: Int
internal var price: Double
init (open: Bool, spotsLeft: Int, price: Double) {
self.open = open
self.spotsLeft = spotsLeft
self.price = price
}
internal func getPrice() -> Double {
return self.price
}
internal func getSpotsLeft() -> Int {
return self.spotsLeft
}
internal func isOpen() -> Bool {
return self.open
}
}
Coding sample (A) is the correct answer. This is due to the completeness of the class. The class has getters for open, spotsLeft, and price which is what the user in the prompt asks for to be able to display them on a website.
Coding sample (B) is incorrect because everything is set to private. If the getter functions are private, then the user cannot use them for the website.
Coding sample (C) is incorrect because there are no return types on the functions. In Swift, the functions must have return types.
Coding sample (D) is incorrect because it is just the shell of a class and does not actual perform any functions.
Example Question #4 : Object Oriented Program Design
USING STATIC VARIABLES
CONSIDER THE FOLLOWING JAVA CODE:
public class Employee
{
static int nextID = 1;
String name;
int employeeID;
Employee(String n){
name = n;
employeeID = nextID;
nextID++;
}
public String getName(){
return name;
}
public int getID(){
return employeeID;
}
public static void main(String[] args)
{
Employee emp3 = new Employee("Kate");
Employee emp1 = new Employee("John");
Employee emp2 = new Employee("Sandy");
System.out.println(emp1.getName() + " ID: " + emp1.getID());
System.out.println(emp3.getName() + " ID: " + emp3.getID());
}
}
WHAT WOULD BE THE CONSOLE OUTPUT?
John ID: 2
Kate ID: 1
John ID: 1
Sandy ID: 2
John ID: 1
Sandy ID: 1
John ID: 1
Kate ID: 2
John ID: 2
Kate ID: 1
Static variables are associated with a class, not objects of that particular class. Since we are using a static class variable "nextID" and assigning that to employeeID as a new employee is created and then increasing it, the class variable is updated for the whole class.
In our code, we have emp3 being created first. This means that Kate is created with an employeeID equaling the current value of nextID (which is 1 in this case). Afterwards, the nextID value increases by 1. Next emp1 is created with the name of John and an employeeID equaling to the current value of nextID (which became 2 due to the increased that occurred when Kate was created). Lastly, emp2 is created with the name of Sandy and an employeeID equaling the current value (at that point in the code) of nextID. Meaning Sandy has an employeID value of 3.
Therefore in the first System.out. print statement when we print emp1, we get: John ID: 2
and in the second System.out. print statement when we print emp3 we get:
Kate ID: 1
Example Question #5 : Object Oriented Program Design
What is the relationship between the class Car and the class TwoDoor in the code snippet below?
class TwoDoor extends Car {
}
TwoDoor is the super class to Car
Car is the super class to TwoDoor
TwoDoor implements Car
Car implements TwoDoor
Car is the super class to TwoDoor
Car is the super class to TwoDoor. Since the class TwoDoor extends the class Car, TwoDoor can use methods and variables from Car by calling super.
The answers that mention implements are incorrect because implementing refers to interfaces. For TwoDoor to implement car, the code would look like this:
class TwoDoor implements Car {
}
Example Question #1 : Object Oriented Program Design
What is the difference between extending and implementing?
You can extend an interface and implement a subclass. Subclasses can be implemented as frequently as needed but you can only extend a single interface.
Extend refers to a relation between a superclass and subclass. Implementing refers to an interface. You can implement only a single interface but you can implement multiple classes.
Extend refers to a relation between a superclass and subclass. Implementing refers to an interface. You can extend only a single class but you can implement multiple interfaces.
Extend refers to a relation between a superclass and subclass. Implementing refers to an interface. You can extend only a single class but you can implement multiple interfaces.
By default, all classes are extensions of the Object class and therefore are extending a single class. Due to that concept, implementation was made with the idea that notion of only being able to extend a single class. Thus they allow you to implement an infinite amount of classes.
Example Question #3 : Class Design
Describe the difference between overloading a method and overriding a method.
Overloading is the same method name, same parameters but the name of the parameter is different. Overwriting is calling the same method in the same class.
Overwriting is the same method name and different parameters. Overloading is rewriting the body of the definition.
Overloading is the same method name and different parameters. Overwriting is rewriting the body of the definition.
Overloaded methods and overridden methods both take in the same methods but overridden methods return a different parameter.
Overloading is the same method name and different parameters. Overwriting is rewriting the body of the definition.
When you override a method, you are completely replacing the functionality of the method by overshadowing the method in the parent child with the one in the child class.
Overloading a method just allows you to have the same method name to have multiple meanings or uses. An example is '+' operator. In languages like Java, you can use '+' to append strings, add doubles, or add integers.
Example Question #2 : Class Design
Which of the following header statements allow you to omit using the std:: when using cout?
#include <iostream>
#include <standard>
#include <cmath>
using namespace std;
You don't need a header statement.
using namespace std;
You must put using namespace std; at the top of your file to avoid having to type std:: every time you use cout and cin.
All Computer Science Resources
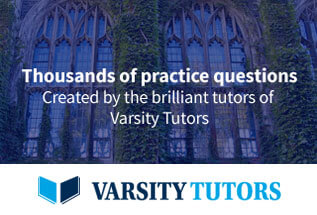