All Computer Science Resources
Example Questions
Example Question #271 : Computer Science
USING FUNCTIONS
Consider the following C++ code to perform the following subtraction:
9 - 6:
#include <iostream>
using namespace std;
int main() {
int total = 0;
cout << "This code performs the following math operation: 9 - 6 = ";
total = sub(6,9);
cout << total;
}
int sub(int num1, int num2) {
return (num2 - num1);
}
Is there anything wrong with the code?
There is nothing wrong with this code.
The function prototype is missing.
The code is missing the math library. It should have
#include
The arguments in total should be flipped. Such as: total = sum(9,6);
The function prototype is missing.
Taking a look at each answer, we can see that the order of the arguments passed in the sub function is correct because of the fact that num1 is being subtracted from num2. Also, for simple math operations there isn't a need to include the math library. Lastly, any operation can be done within a function or in main; there is no wrong method however, creating and using functions makes the code in main() easier to read and cleaner.
Now within main(), when the sub() function is called the function has not been defined; meaning that it is not within the scope. This means that we should place the function prototype before main() so that when the function is called, the compiler know that there's a function called sub() that takes in two integer parameters and returns an integer. Therefore this addition will fix our scope issue.
#include <iostream>
using namespace std;
int sub(int num1, int num2); //This addition is the function prototype
int main() {
int total = 0;
cout << "This code performs the following math operation: 9 - 6 = ";
total = sub(6,9);
cout << total;
}
int sub(int num1, int num2) {
return (num2 - num1);
}
Note: Another fix to this issue, is placing the functions right before main(). Although this method is not particularly recommended because it makes main() harder to find, people still use it. The following is an example of fixing the issue by placing the function before main(). Note that the function prototype is not needed when using this method:
#include <iostream>
using namespace std;
int sub(int num1, int num2) {
return (num2 - num1);
}
int main() {
int total = 0;
cout << "This code performs the following math operation: 9 - 6 = ";
total = sub(6,9);
cout << total;
}
Example Question #1 : Post Conditions
double square(double n){
return n*n;
}
What MUST be true immediately after the above code snippet has run?
The result will be a negative number.
The value of the input parameter changes.
It is impossible to tell.
The result will be stored in a new variable.
The result will be a positive number.
The result will be a positive number.
Squaring a real number will always produce a positive number. The result does not have to be stored in a new variable; it could be a value that is only needed for a one-off expression, thus, not worthy to be stored in memory. Lastly, since the input was passed by value and not by reference, its initial value will stay the same.
Example Question #2 : Program Correctness
Which of the following code excerpts would output "10"?
int num = 10;
num = (num > 0) ? 11: 12;
System.out.println(num);
int num = 5;
num = (num > 0) ? 10: 11;
System.out.println(num);
int num = 5;
num = (num < 0) ? 10: 11;
System.out.println(num);
None of the answers are correct.
int num = 5;
num = (num > 0) ? 11: 10;
System.out.println(num);
int num = 5;
num = (num > 0) ? 10: 11;
System.out.println(num);
Each bit of code has something similar to this:
num = (boolean statement) ? X : Y;
The bit at the end with the ? and : is called a ternary operator. A ternary operator is a way of condensing an if-else statement. A ternary operator works like this:
<boolean statement> ? <do this if true> : <do this if false>
The correct answer is
int num = 5;
num = (num > 0) ? 10: 11;
System.out.println(num);
Therefore, the ternary operator portion of code, when converted to an if-else, looks like this:
if (num > 0) {
num = 10;
else {
num = 11;
}
num
is 5, which is greater than 0, it would go into the if
, so num
would then get 10. Then, num
gets printed, which means 10 gets printed (the correct answer).Example Question #3 : Program Correctness
True or False.
The assertion in this code snippet is to prevent users from inputting bad data.
public class UserInput {
int userInput;
public static void main(String[] args) {
assertTrue(isInteger(args[0]));
userInput = args[0];
userInput = 25 - userInput;
}
}
True
False
True
The assertion is used to validate user input. The user is able to input anything into the value at args[0]. Since this is the case, we must validate and make sure that the user is inputting what we want. To make this code snippet better, add error checking to do something if the user did not input an integer.
Certified Tutor
Certified Tutor
All Computer Science Resources
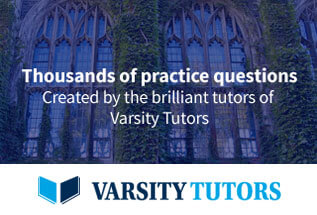