All Computer Science Resources
Example Questions
Example Question #21 : Standard Operations & Algorithms
Which of the following defines a method that successfully deletes an item from an array of integers?
public static int[] del(int[] a,int delIndex) {
if(a == null || delIndex < 0 || delIndex >= a.length) {
return null;
}
for(int i = 0; i <= delIndex; i++) {
if(i == delIndex) {
delete a[i];
break;
}
}
return a;
}
public static int[] del(int[] a,int delIndex) {
if(a == null || delIndex < 0 || delIndex >= a.length) {
return null;
}
int[] ret = new int[a.length - 1];
for(int i = 0; i <= delIndex; i++) {
ret[i] = a[i];
}
return ret;
}
public static int[] del(int[] a,int delIndex) {
if(a == null || delIndex < 0 || delIndex >= a.length) {
return null;
}
int[] ret = new int[a.length - 1];
for(int i1=0,i2 = 0; i1 < a.length; i1++) {
if(i1 == delIndex) {
delete a[i1];
}
}
return ret;
}
None of these work correctly
public static int[] del(int[] a,int delIndex) {
if(a == null || delIndex < 0 || delIndex >= a.length) {
return null;
}
int[] ret = new int[a.length - 1];
for(int i1=0,i2 = 0; i1 < a.length; i1++) {
if(i1 != delIndex) {
ret[i2] = a[i1];
i2++;
}
}
return ret;
}
public static int[] del(int[] a,int delIndex) {
if(a == null || delIndex < 0 || delIndex >= a.length) {
return null;
}
int[] ret = new int[a.length - 1];
for(int i1=0,i2 = 0; i1 < a.length; i1++) {
if(i1 != delIndex) {
ret[i2] = a[i1];
i2++;
}
}
return ret;
}
Of course, this is an inefficient way to do such a delete, but arrays are rather "locked" data structures in that their size cannot change without a reassignment. (You could, of course keep track of the last "used" index. However, that is a different implementation, not reflected here.) The correct answer is the one that carefully goes through the original array, copying those contents into the new array skipping the one value that is not wanted.
Example Question #21 : Standard Operations & Algorithms
public static boolean remove(int[] arr, int val) {
boolean found = false;
int i;
for(i = 0; i < arr.length && !found; i++) {
if(arr[i] == val) {
found = true;
}
}
if(found) {
for(int j = i; j < arr.length;j++) {
arr[j - 1] = arr[j];
}
arr[arr.length - 1] = 0;
}
return found;
}
For the code above, what will be the content of the variable arr
at the end of execution, if the method is called with the following values for its parameters:
arr = {3,4,4,5,17,4,3,1}
val = 4
{3, 5, 17, 3, 1, 0, 0, 0}
None of the other answers
{3, 4, 5, 17, 4, 3, 1, 0}
{3, 5, 17, 3, 1}
{3, 4, 5, 17, 4, 3, 1}
{3, 4, 5, 17, 4, 3, 1, 0}
This code simulates the removal of a value from an array by shifting all of the elements after that one so that the array no longer contains the first instance of that value. So, for instance, this code takes the original array {3,4,4,5,17,4,3,1}
and notices the first instance of 4: {3,4,4,5,17,4,3,1}
. Next, it starts shifting things to the left. Thus, some of the steps will look like this:
{3,4,4,5,17,4,3,1}
{3,4,5,5,17,4,3,1}
{3,4,5,17,17,4,3,1}
...
{3,4,5,5,17,4,1,1}
Then, at the very end, it sets the last element to 0:
{3,4,5,17,4,3,1,0}
Example Question #21 : Computer Science
int[] arr = new int[20];
int x = 6,i=2;
for(int j = 0; j < x; j++) {
arr[j] = j * 40 + 20;
}
for(int j = x; j > i; j--) {
arr[j] = arr[j - 1];
}
arr[i] = 20;
for(int j = 0; j < x; j++) {
System.out.print(arr[j] + " ");
}
What is the output for the code above?
20 60 20 100 140 180
None of the others
20 60 20 100 140 180 220
20 60 20 100 100 100
20 60 20 140 180 220
20 60 20 100 140 180
It is easiest to understand this by a bit of code parsing:
First, there is the loop: for(int j = 0; j < x; j++) { // ...
This will fill the array with 20 + 0, 20 + 40, 20 + 80, ... Thus, it will be:
20, 60, 100, 140, 180, 220
Next, there is the array: for(int j = x; j > i; j--) { // ...
This basically shifts everything after index i backward by 1. Thus you have:
20, 60, 100,100, 140, 180, 220
Next, you set arr[2] equal to 20:
20, 60, 20,100, 140, 180, 220
Finally you output each of the first 6 elements. Be careful here. Notice that it is from j = 0 to x - 1!
Thus, the answer is:
20 60 20 100 140 180
This algorithm basically implements a simple kind of array deletion.
All Computer Science Resources
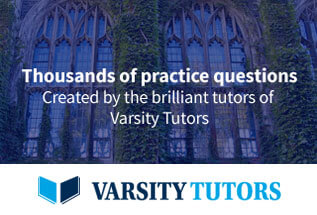