All Computer Science Resources
Example Questions
Example Question #141 : Computer Science
int foo[] = {1, 2, 3, 4, 5};
number = 100 + foo[4];
What is the value of number
?
Error.
The answer is 105 because arrays are zero indexed. This means that the first position has the subscript 0, the second subscript 1, and so on. 5 is in the fifth space, located at subscript 4. We would access it by saying foo[4]. As another example, if we wanted to access one, we would say foo[0].
Example Question #1 : Arrays
Given the following in C++:
int * data = new int[12];
Pick an expression that is equivalent to : data[5];
data + 5
(data + 5)&
(data+5)*
&(data+5)
*(data +5);
*(data +5);
Let's look at this line of code:
int * data = new int[12]
An int pointer is created and an array of size 12 is assigned to it.
When data[5] is called, the "data" is dereferenced and the value of the 6th position is returned.
The only one of the choices that does this is
*(data + 5)
In line above, the pointer is incremented ot the 6th position and is then dereferenced to get the value.
If the code was
*data
The first item at the first position will be returned.
*(data+1)
This will return the item and the second position and so on.
Example Question #1 : Arrays
TWO DIMENSIONAL ARRAYS
Given the following initialized array:
int fourth;
int[][] myArray = { {1, 2, 3},
{4, 5, 6},
{7, 8, 9} };
Using myArray, how can I store in variable "fourth", the number 4?
fourth = myArray[2][1];
fourth = myArray[1][1];
fourth = myArray[0][1];
fourth = myArray[1][0];
fourth = myArray[1][0];
When a two dimensional array is created and initialized, the way to access the items inside the matrix is by calling the array with the row and column (i.e. myArray[ROW][COLUMN]). Keeping in mind that arrays start at 0, the number four would be in row 1, column 0. Therefore to save that number into the variable "fourth" we'll do the following:
fourth = myArray[1][0];
*Note: myArray[1][0] is not the same as myArray[0][1].
myArray[1][0] =4 because it is the item located at row=1 and column = 0.
myArray[0][1] =12 because it is the item located at row=0 and column = 1.
Example Question #11 : Program Design
True or False.
The best data structure to represent a set of keys and values is an array.
False
True
False
Arrays can be two-dimensional. However, when trying to keep track of keys and values it can become complicated when using an array. HashMaps are the best way to represent data containing keys and values.
Example Question #151 : Computer Science
Define an unwrapped integer array in Swift (iOS).
var arr: Int = []
var arr: [Int] = []
var arr = [Int]
var arr = []
var arr: [Int] = []
In Swift, the variable must be declared first with var then given a name. So now we have var arr then to unwrap, we must add a type var arr: [Int] and then initialize. Therefore, we have var arr: [Int] = []. var arr = [] is technically correct, but the prompt asks you to unwrap the variable.
Example Question #152 : Computer Science
Define an unwrapped string array in Swift (iOS).
var arr: [String] = []
var arr: String = []
var arr = [String]
var arr = []
var arr: [String] = []
In Swift, the variable must be declared first with var then given a name. So now we have var arr then to unwrap, we must add a type var arr: [String] and then initialize. Therefore, we have var arr: [String] = []. var arr = [] is technically correct, but the prompt asks you to unwrap the variable.
Example Question #41 : Common Data Structures
Which of these instantiate a matrix called matrx with 5 columns and 4 rows that takes in integers?
int [] [] matrx = new int [] [5];
int [] [] matrx = new int [5] [];
int [] [] matrx = new int [4] [5];
int [] [] matrx = new int [5] [4];
int [] [] matrx = new int [] [4];
int [] [] matrx = new int [] [4];
You create a matrix also known as a 2 dimensional array the same way you'd instantiate a normal array except the first array space remains blank and you'd insert the number for the amount of rows. Due to the fact that you want 5 columns and 4 rows, you'd only input the 4 into the second array.
All Computer Science Resources
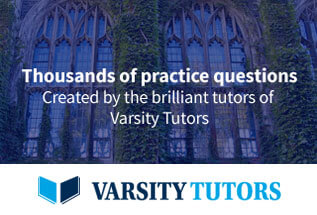