All Computer Science Resources
Example Questions
Example Question #1 : Insertions
public static int[] doWork(int[] arr, int val,int index) {
int[] ret = new int[arr.length + 1];
for(int i = 0; i < index; i++) {
ret[i] = arr[i];
}
ret[index] = val;
for(int i = index + 1; i < ret.length; i++) {
ret[i] = arr[i - 1];
}
return ret;
}
What does the code above perform?
It searches for a value in the array, storing a new value at the index at which that value was found
It deletes a value from the array, so long as it is at a given index
It inserts a new value into an array by overwriting the value that was there before
It inserts a new value in to an array, adding that value to the existing array
None of the others answers
It inserts a new value in to an array, adding that value to the existing array
A quesiton like this is most easily understood by doing a careful reading of the code in question. Let's consider each major section of code:
int[] ret = new int[arr.length + 1];
This line of code creates a new array, one that is 1 longer than the array arr
.
for(int i = 0; i < index; i++) . . .
This loop copies into ret
the values of arr
up to index - 1
.
(This is because of the i < index
condition)
Then, the code stores the value val
in ret[index]
for(int i = index + 1; i < ret.length; i++) . . .
It then finishes copying the values into ret
.
Thus, what this does is insert a value into the original array arr
, returning the new array that is one size larger. (This is necessary because of the static sizing of arrays in Java.)
Example Question #1 : Insertions
public static int[] doWork(int[] arr, int val,int index) {
int[] ret = new int[arr.length + 1];
for(int i = 0; i < index; i++) {
ret[i] = arr[i];
}
ret[index] = val;
for(int i = index + 1; i < ret.length; i++) {
ret[i] = arr[i - 1];
}
return ret;
}
Which of the following is a possible error in the first loop in code above?
I. The array arr
might be indexed out of bounds.
II. The array ret
might be indexed out of bounds.
III. A null pointer exception might occur.
Both II and III
Only I
I , II, and III
Only III
Both I and III
Both I and III
The most obvious possible error is that the array arr might be a null value. You need to check for these kinds of values before using the variables. (If you do arr[0]
on a null value, an exception will be thrown.) In addition, it is possible that a value for index might be given that is too large. Consider if index = 100
but arr
is only 4 elements long. Then, you will have ret
be a 5 value array. When the first loop starts to run, you will go all the way to 99 (or at least attempt to do so) for the index value i
; however, once you get to ret[4] = arr[4]
, there will be an out of bounds error on arr
, which only has indices 0, 1, 2, and 3. Of course, there could be other problems later on with ret
, but the question only asks about this first loop.
Example Question #11 : Computer Science
int[] arr = {0,0,0,0,0,0,0,0,0,0};
int arrFill = 0;
int val;
// In here, n items are added. ArrFill is n. Presume that n <= 9
// The variable val now contains a new value to be added
Which of the following blocks of code push a new value into arr as though it were a stack?
arr[arrFill + 1] = val;
arrFill++;
arr[arrFill] = val;
arrFill++;
None of the others
arr[arrFill - 1] = val;
arrFill++;
arr[0] = val;
arrFill++;
arr[arrFill] = val;
arrFill++;
Let us presume that the array arr looks like the following list of integers:
{4,51,41,0,0,0,0,0,0,0}
Presume that our new value is 77.
A stack could either push on to the beginning and move everything back one, giving you something like:
{77,4,51,41,0,0,0,0,0,0}
However, none of the options do this. (No, not even the one that uses the index 0. This one does not add on to the array so much as replace the first element of it.)
So, the other option is to put it on the "end" of the list. The variable arrFill is being used for this purpose. If it is 3, this means that it is the value of the fourth element. Thus, you can set arr[4] = 77 (where 4 really is arrFill).
This will give you:
{4,51,41,77,0,0,0,0,0,0}
You also need to add one to the value of arrFill.
The other options do not correctly address the array. They either are too large or too small by one.
Example Question #1 : Insertions
True or False.
The worst case for insertion into an ArrayList is O(N).
True
False
True
Insertion into an ArrayList is typically O(1). However, since an ArrayList uses an array as its underlying data structure, there can be a need to resize the underlying array. Resizing an array requires creating a new array and copying all the data from the old array to the new array which takes O(N) time.
Certified Tutor
All Computer Science Resources
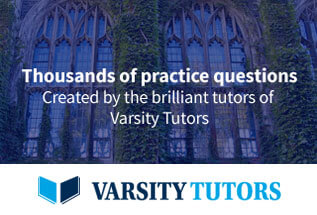