All Computer Science Resources
Example Questions
Example Question #1 : Evaluating Boolean Expressions
int currentYear = 2016;
bool leapYear;
if (currentYear % 4 == 0) {
leapYear = true;
} else {
leapYear = false;
}
febDays = leapYear ? 28 : 29
Based on the code above, what value will febDays
have?
The ternary operator works as follows:
(condition to be checked) ? (value to return if true) : (value to return if false)
The answer is purposely logically incorrect to force you to consider the structure of the code. While 2016 is obviously a leap year where February should have 29 days, the ternary operator used is set to return 28 days if true and 29 if false. So in this case, febDays
will be 28.
Example Question #62 : Primitive Data Types
What does the following code print?
int i = 3;
int j = 4;
int k = 5;
int l = i * k - j;
int m = i*2 + j + k;
if ( ( l > m ) || ( l * i ) = (m+m+i) )
System.out.println("The statement proves true");
else
System.out.println("The statement proves false");
Runtime Error
The statement proves false.
Compiler Error
The statement proves true.
Compiler Error
In the second condition, it is not a statement of equivalence but a statement of assignment. This would cause it to have a runtime error. If it was to be a == instead of =, the statement would be true.
Certified Tutor
Certified Tutor
All Computer Science Resources
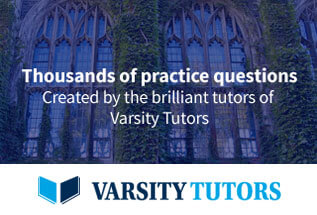