All Computer Science Resources
Example Questions
Example Question #191 : Computer Science
What will the following code result in?
int main(){
int i = 7;
const int * ip = &i;
cout<< *ip << endl;
}
Runtime Error
7
Compilation error
None of the above.
8
7
Let's take a look at the code.
int i = 7;
This line assigns 7 to the variable "i".
const int * ip = &i;
This line creates a constant int pointer and assigns the address of "i" to the pointer.
cout << *ip << endl;
This line prints the dereference of the pointer, which holds the value 7.
Example Question #31 : Programming Constructs
class Base{
public:
void foo(int n) { cout << "Base::foo(int)\n"; }
};
class Derive: public Base{
public:
void foo(double n) { cout << "Derived::foo(double)\n";}
};
int main(){
Derived der;
der.foo(42);
}
The above code is written in C++, what is the output of the program?
Derived::foo(double).
Base::foo(int).
None of the above.
The program does not generate any output.
The program does not compile.
Derived::foo(double).
This is an example of inheritance. The child class (Derived) is an inherited class of the parent class (Base). When the Derived object is created and the "foo" method is called, foo in the Derived class will be called. If there is the same method in the parent class and child class, the child's method will be called.
Example Question #1 : Console Output
In Swift (iOS), give a description of what this method does.
func getDivisors(num: Int, divisor: Int) -> Bool {
var result: Bool = False
if (num % divisor == 0) {
result = True
}
println(result)
}
This function doesn't do anything.
This function returns false if the number is evenly divisible by the divisor. It returns true otherwise.
This method returns true if the number is evenly divisible by the divisor. It returns false otherwise.
This function returns true or false.
This method returns true if the number is evenly divisible by the divisor. It returns false otherwise.
The modulus function "%" determines the remainder of a division. If I have 1 % 2, the remainder is 1. If I have 2 % 2, the remainder is 0. Therefore, if the remainder is equal to 0, then the number is divisible by the function. Thus, the method returns true if the number is evenly divisble by the divisor and false otherwise.
Example Question #2 : Console Output
Does the code compile and if yes, what is the the output?
public class Test
{
public static void main ( String [] args )
{
int x = 2;
if (x = 2)
System.out.println("Good job.");
else
System.out.println("Bad job);
}
}
Yes it compiles and it prints good job.
Yes it compiles and it prints bad job.
No, it doesn't compile.
No, it doesn't compile.
It doesn't compile. It is supposed to be x == 2 within the if statement not x = 2. The if statement checks for booleans (only true or false) and cannot convert an integer into a boolean to meet that check.
All Computer Science Resources
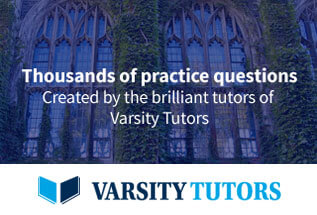