All Computer Science Resources
Example Questions
Example Question #1 : Class Inheritance
class Pet {
public:
Pet() {}
virtual void bar() {cout << "In pet bar(); }
};
class Cat : public Pet {
public:
virtual void eat() {cout << "Cat eating"; }
virtual void bar() {cout << "In Cat bar()"; }
};
Given the above classes, what would the result of:
int main(){
Cat felix;
Pet peeve;
peeve = felix;
peeve.bar();
}
Runtime error
Compilation Error
The program runs and prints: In Cat bar()
The program runs and prints: In Pet Bar()
None of the above
The program runs and prints: In Pet Bar()
The Cat class is inherited from the Pet class and an instance of each object is created in main.
peeve = felix;
When felix is assigned to peeve, it loses it's atributes as a Cat and now becomes a Pet. When the bar method is called, it will call bar inside the Pet class and not the Cat class.
If the line of code was:
felix = peeve;
Then, the program will crash. This crashes because we are trying to assign a Pet (which is the parent), to a Cat (which is a child class). Assigning a parent to a child will cause a Slicing Problem because the parent is "bigger" than the child. If you try to put something big (a Pet) into a small container (a Cat), it will not work.
Example Question #1 : Class Inheritance
class Member{
public:
Member() { cout<< 1 ; }
};
class Base{
public:
Base(){ cout<< 1; }
Memeber member;
};
class Derived : public Base{
public:
Derived(){ cout << 3; }
};
int main(){
Derived der;
}
Given the code above, what is the output of the program?
312
321
213
123
132
123
When an object is created the order of which everything is created is as follows:
1. Member variables are created first.
2. The constructor of the parent class called (if the object has a parent)
3. The object's own constructor is called.
Following the code, we can see that a Derived object is created in main. Since the Derived class has a parent, the call order jumps to the Base class. Now we are inside the Base class, nothing has been outputted yet. Inside the Base class, the local variables are created first. This brings us to the Member class.
In the Member class, the constructor is called and 1 is outputted.
After this, we jump back to the constructor of the Base class and 2 is outputted.
Finally, we get back to where we started, the Derived class. The constructor of derived is called and 3 is outputted. The final output is 123.
Example Question #2 : Class Inheritance
Consider the following code:
public class Rectangle {
private double width, height;
public Rectangle(double w,double h) {
width = w;
height = h;
}
public double getArea() {
return width * height;
}
public double getPerimeter() {
return 2 * width + 2 * height;
}
}
public class Square {
private double side;
public Square(double s) {
side = s;
}
public double getArea() {
return side * side;
}
public double getPerimeter() {
return 4 * side;
}
}
Which of the following represents a redefinition of Square that utilizes the benefits of inheritance?
public class Square implements Rectangle {
public Square(double s) {
super(s,s);
}
}
public class Square extends Rectangle {
private double side;
public Square(double s) {
super(s,s);
}
}
public class Square extends Rectangle {
public Square(double s) {
new Rectangle(s,s);
}
}
public class Square extends Rectangle {
public Square(double s) {
super(s,s);
}
}
public class Square {
private Rectangle r;
public Square(double s) {
r = new Rectangle(s,s);
}
public double getArea() {
return r.getArea();
}
public double getPerimeter() {
return r.getPerimeter();
}
}
public class Square extends Rectangle {
public Square(double s) {
super(s,s);
}
}
We know that a square really is just a subclass of a rectangle, for it is merely a rectangle having four sides that are all equal. Using inheritance, you can very easily reuse much of your Rectangle
code. First, you need to extend the Rectangle
class:
public class Square extends Rectangle { . . .
Next, you can be rid of the field side
. This allows you to alter the constructor for Square
to call the Rectangle
constructor. You do this using super
(because Rectangle is the superclass).
After this, you can delete the getArea
and getPerimeter
methods, for they will be handled by the superclass. This gives you a very simple bit of code!
Example Question #1 : Class Inheritance
Which of the following is TRUE about the Object class?
Object inherits a minimum of four classes.
Every class has all of Object's methods.
Object has one superclass, Entity.
Object is the only class that never has any descendants.
None of these answers are true.
Every class has all of Object's methods.
Object is the most basic class which all others, even user made ones, inherit. Therefore, all classes have Object's methods. A way to think of classes is to think of a tree: the Object class is the lowest node on the tree, where all other nodes can connect back to.
Example Question #5 : Class Inheritance
True or False.
The class BetterMan inherits from the class Man.
public class BetterMan extends Man {
}
False
True
True
The class BetterMan inherits methods from the class man. The keyword "extends" means that BetterMan will get all the methods from Man plus be able to extend the class by adding its own methods. All methods from Man can be used in BetterMan by calling the keyword better.
Example Question #301 : Computer Science
What does the code print?
class
Parent{ final
public
void
show() {
System.out.println(
"Parent::show() called"
);
}
}
class
Child
extends
Parent {
public
void
show() {
System.out.println(
"Child::show() called"
);
}
}
public
class
Main {
public
static
void
main(String[] args) {
Parent parent
=
new
Child();
parent
.show();
}
}
Parent::show() called
Child::show() called
Runtime Error
Child::show() called
Parent::show() called
Compiler Error
Compiler Error
Final methods can't be overriden. So the code won't compile because of this. Now if the final modifier were to be removed. The code would compile and run and produce:
Child::show()
Example Question #301 : Computer Science
class Pet {
public:
Pet() {}
virtual void bar() {cout << "In pet bar(); }
};
class Cat : public Pet {
public:
virtual void eat() {cout << "Cat eating"; }
virtual void bar() {cout << "In Cat bar()"; }
};
Given the above classes, what would the result of:
int main(){
Pet * petPtr = new Cat();
petPtr -> eat();
}
The program compiles but has a runtime error
None of these
The program runs and prints: "Cat eating"
Compilation error
The program runs and prints: "Pet eating"
Compilation error
When a child class inherits the properties of a parent class, some attributes are inherited and some aren't. In C++, the constructor of the parent class is not inherited. The Cat class does not have a constructor therefore the program will not compile.
All Computer Science Resources
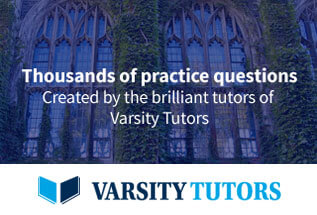