All AP Computer Science A Resources
Example Questions
Example Question #1 : Testing
The function fun
is defined as follows:
public int fun(int[] a)
{
a[a.length - 1] = a[0];
return a[0] + (a[0] % 2);
}
a[0]
after the following code segment is executed?int[] a = {3, 6, 9, 12};
a[0] = fun(a);
12
13
3
6
9
12
The first part of fun
assigns the value of the last location of the array to the first location. Then, it returns the a[0] + (a[0] % 2)
. That last portion will be a "1
" if a[0]
is odd, and "0
" if a[0]
is even. Since a[0] == 12
, which is even, the expression evaluates to 12 + 0
, which is 12
.
Example Question #1 : Unit Testing
x
, y
, and z
after the following code is executed?int x = 4, y = 3, z;
for (int i = 0; i < 5; i++)
{
z = x + y;
y = x - y;
x = z;
}
x == 28, y == 4, z== 4
x == 16, y == 12, z== 16
x == 32, y == 24, z == 32
x == 28, y == 4, z== 28
x == 14, y == 2, z == 14
x == 28, y == 4, z== 28
The loop will run 5 times. The values of x
, y
, and z
after each run will be as follows:
i == 0: x == 7, y == 1, z == 7
i == 1: x == 8, y == 6, z == 8
i == 2: x == 14, y == 2, z == 14
i == 3: x == 16, y == 12, z == 16
i == 4: x == 28, y == 4, z == 28
At the end, i
will equal 5, and the values will no longer change.
Example Question #2 : Testing
if (s.length() <= 5)
return s5 + s4 + s3 + s2 + s1;
else
return s1 + s2 + mystery(s3) + s4 + s5;
}
What is the output of
System.out.println(mystery("ABNORMALITIES"));
None of these answers is correct.
SEITILAMRONBA
SEITRMALIONBA
ABNOILAMRTIES
ABNORMALITIES
ABNOILAMRTIES
The .substring()
method takes the character at the first number in the arguments, and goes through the String until it reaches the second number in the arguments, without copying the character at the second number.
In the first part of mystery()
, the Strings s1, s2, s3, s4, and s5 are made and filled. If n = # of characters in s, s1 gets the first character in s, s2 gets the second character in s, s3 gets the third through n-2 characters, s4 gets the n-1 character, and s5 gets the last character.
Let's look at the second portion of mystery().
if (s.length() <= 5)
return s5 + s4 + s3 + s2 + s1;
else
return s1 + s2 + mystery(s3) + s4 + s5;
The if
statement checks the length of s, and if it's less than or equal to 5, it returns a String made from s5, followed by s4, etc. If s were equal to "abcde", then the if
would evaluate to true, and would return "edcba". In recursion, this is known as the "base case".
The else
statement is for strings that are greater than 5 characters in length. It returns s1, followed by s2, then the result of mystery(s3), then s4 and s5. The fact that it calls itself makes this recursion.
Let's step through the example. The argument for mystery()
, s, is "ABNORMALITIES". After the first part, this is the result:
else
, so it returns the following:A + B + mystery(NORMALITI) + E + S
else
, so it returns the following:N + O + mystery(RMALI) + T + I
A + B + N + O + mystery(RMALI) + T + I + E + S
if
this time. It returns the following:I + L + A + M + R
A + B + N + O + I + L + A + M + R + T + I + E + S
Example Question #2 : Unit Testing
Consider the Array
int[] arr = {1, 2, 3, 4, 5};
What are the values in arr
after the following code is executed?
for (int i = 0; i < arr.length - 2; i++)
{
int temp = arr[i];
arr[i] = arr[i+1];
arr[i+1] = temp;
}
OutOfBoundsException
15432
12345
23451
54321
23451
We start with an array, arr,
of size 5, containing {1, 2, 3, 4, 5}.
The loop in the code,
for (int i = 0; i < arr.length - 2; i++)
loops through the array up to the second to last cell, given that arr.length - 2
is the index of the second to last cell, and it starts at the first cell.
Let's look at the code inside the loop.
int temp = arr[i];
arr[i] = arr[i+1];
arr[i+1] = temp;
arr[0] == 1
arr[1] == 2
temp = 1
arr[0] = 2
arr[1] = 1
arr[] == {2, 1, 3, 4, 5}
arr[1] == 1
arr[2] == 3
temp = 1
arr[1] = 3
arr[2] = 1
arr[] == {2, 3, 1, 4, 5}
When i = 2
arr[2] == 1
arr[3] == 4
temp = 1
arr[2] = 4
arr[3] = 1
arr[] == {2, 3, 4, 1, 5}
arr[3] == 1
arr[4] == 3
temp = 1
arr[3] = 3
arr[4] = 1
arr[] == {2, 3, 4, 5, 1}
As the loop progresses, it moves whatever value is in arr[0]
all the way to the end of the array.
All AP Computer Science A Resources
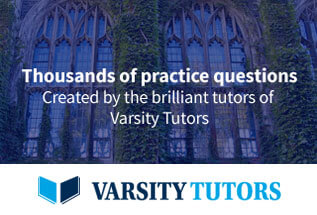