All Computer Science Resources
Example Questions
Example Question #232 : Computer Science
Given a vector of ints called "intVec", write a "ranged for" loop, also sometimes known as a "for each" loop, to double the values fof all elements in a vector. (In C++)
for( int& i : intVec(){
i * = 2;
}
for( int& i : intVec(){
i * 2;
}
for( int i = 0; i < intVec.size(); ++i){
intVec[i] *= 2;
}
for( int i : intVec(){
i * = 2;
}
for( int i = 0; i < intVec.size(); ++i){
intVec[i] * 2;
}
for( int& i : intVec(){
i * = 2;
}
A "for each" loop was introduced in C++ 11 which allows the user to loops through a container of items without writing a traditional loop. Let's take a look at all the choices.
for( int i : intVec(){
i * = 2;
}
Although this looks like the correct answer, it's not. The int i in the first part of the for loop is passed in "by value", which means that a copy of intVec will be made and the integers inside the copy will be doubled. The integers inside the original intVec vector will be unaffected. To fix this problem, int i needs to be passed into the for loop "by reference", which is done by adding a "&" symbol after "int".
The correct loop is:
for( int& i : intVec(){
i * = 2;
}
Let's take a look at the other choices:
for( int& i : intVec(){
i = 2;
}
This one will not work because it is setting all items in intVec to 2, not doubling them.
All the other for loops are not "for each" loops so they are incorrect even if they accomplish the same output.
All Computer Science Resources
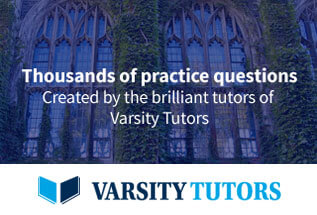