All AP Computer Science A Resources
Example Questions
Example Question #11 : Evaluating Boolean Expressions
Which is equivalent?
int a = 5
int b = 10
int c = 25
int d = 15
- (a + b) == c
- (a * b) == d
- (a + b) == d
- (a * c) == b
4
2
3
1
3
3 is the correct answer because a = 5 and b = 10 so 5 + 10 = 15 which is d.
Example Question #61 : Primitive Data Types
Which is true?
a = 4
b = 2
c = 5
d = 6
a) a = c - d
b) a = b - d
c) a = d - b
d) a = c * d
a)
d)
c)
b)
c)
c) is the correct choice because a = 6 - 2 since d = 6 and b = 2. Substitute the numbers for the variables in each answer choice. You will see that all of the answer choices are not equal to 4 except for c).
Example Question #62 : Primitive Data Types
True or False.
This code snippet returns an error.
String s1 = "foo";
String s2 = "foo";
return s1 == s2;
True
False
False
While the code snippet doesn't return an error, it also doesn't return the answer that you want. Testing string equality requires the method String.equals(). This code snippet uses == to compare two strings. == is a pointer equality function. To get the expected answer (true), the code should return s1.equals(s2).
Example Question #61 : Primitive Data Types
Which of the following Boolean expression evalutes to True?
I. False && False && False && True && False
II. (False && False) || (True || False) || (False && (True || False))
III. (True && False) && (True || False || (True && True))
IV. !(True && True && False)
V. False || False || False || True || False
I, IV, V
II, IV, V
III, IV, V,
IV, V
IV
II, IV, V
Answer: II, IV, V
The best way to approach this problem is to divide each conditional into chunks. Then, if there is at least one True in a long list of OR statements, the statement must be true according to Boolean Logic. Likewise, if there is at least one False in a long list of AND's, the statment must be False. For example
False && False && False && True evalulates to False
and
True || False || False ... evaluetes to True
Statement I evalutes to False because False and any number of And's must evaluate to false because False && X evalutes to False, regardless of the value of X.
Statement II evalutes to True because there is one True statement in a list of OR's that make the entire statement True.
Statement III evalutes to False for the same reason Statement I does.
Statement IV is true because although the conditionals in the parentheses are False, the ! (not) operator negates the False to True.
Statement V is true.
All AP Computer Science A Resources
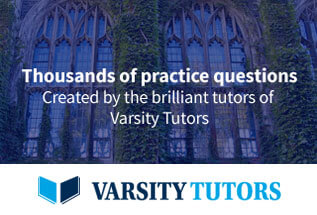