All AP Computer Science A Resources
Example Questions
Example Question #23 : Programming Constructs
1. class test
2. {
3. public:
4. test();
5. exams(int);
6. quiz(int);
7. private:
8. int grade;
9. }
Which line represents the constructor?
1
3
4
8
6
4
The constructor looks like a function declaration but always has the same name of the class.
Example Question #2 : Method Declarations
Fill in the blank.
A ______ function does not specify a return type, but may have any number of inputs.
double
empty
constructor
void
int
void
Remember, a void function does not need a return type. The void keyword tells the program not to expect a return statement. A void function does not necessarily mean that the inputs are void as well. A void function can still have any number of inputs of any type.
Example Question #3 : Method Declarations
Write the stub for a public method named writeToConsole that takes in a boolean b and a string s that returns an integer.
public int writeToConsole()
public int writeToConsole(boolean b, string s)
public writeToConsole(boolean b, string s)
public void writeToConsole(boolean b, string s)
public int writeToConsole(boolean b, string s)
Public methods are prefixed with the word public. The second word is the return type (i.e. int). The third is the name of the method and inside the parentheses goes the inputs to the method (i.e. boolean b and string s).
Example Question #22 : Programming Constructs
True or False.
This code snippet defines a method that takes in two strings as input and will return a boolean as a result.
public void returnBoolean (String input1, String input2);
True
False
False
The prompt specifies that the return type of the method is a boolean. The return type of the method in the code snippet is void. Therefore, it will not return anything. The two input types however are correct.
Example Question #1 : Parameter Declarations
CONSTRUCTORS
Consider the following Java code:
class Person
{
String firstName;
String lastName;
int age;
Person(String l, String f, int a)
{
firstName = f;
lastName = l;
age = a;
}
}
In main, how can I correctly create a person who is 21 years old, whose first name is Indiana, and whose last name is Jones?
Person person1 = new Person();
Person person1 = new Person("Jones", "Indiana", 21);
Person person1 = new Person("Indiana", "Jones", 21);
Person person1 = new Person("Jones", "Indiana", "21");
Person person1 = new Person("Jones", "Indiana", 21);
When we take a look at the constructor, first we notice that it has three parameters. Since there isn't any constructor overloading, this means that we can only create a person if we provide all of the arguments. So we can eliminate Person person1 = new Person() because that answer is not providing any arguments.
Now, we are supposed to be providing first two String arguments and then an integer. Therefore, we can eliminate the following two answers:
Person person1 = new Person("Jones", "Indiana", "21");
Person person1 = new Person(21, "Indiana", "Jones");
We can eliminate the first because the number 21 is being passed as a String instead of an int. It's being passed as a String because it has the double quotation. We can also eliminate the second because an integer is being passed as the first argument, instead of the third.
Lastly whenever a new object is created, the order of the arguments matter! Notice that the constructor is assigning the first parameter "l" to lastName. Therefore, our first argument should be "Jones". Next, the constructor takes the second parameter "f" and assigns it to the variable firstName. This means that our second argument should be "Indiana". Lastly, the constructor takes the third parameter "a" and assigns it to the integer variable age. This means that the last argument we should pass is the number 21 (without any quotes). This means that the correct answer is:
Person person1 = new Person("Jones", "Indiana", 21);
Example Question #1 : Parameter Declarations
Create the method stub for a void method called ingredients that takes in spaghetti, sauce, and meatballs as parameters where the parameters are all strings.
void ingredients(String spaghetti, String sauce, String meatballs);
void ingredients(spaghetti, sauce, meatballs);
ingredients(String spaghetti, String sauce, String meatballs);
ingredients(spaghetti, sauce, meatballs);
void ingredients(String spaghetti, String sauce, String meatballs);
The answer choice is correct because it defines a void method (void ingredients) that takes in the three string parameters (String spaghetti, String sauce, String meatballs). The other answer choices are missing either the void type on the method stub or the type of parameter. Note both the return type of the method (void in this example) and the types of the parameters are very important.
Example Question #32 : Program Implementation
What keyword is needed to create a class variable?
final
private
abstract
static
static
The static modifier makes the variable visible to the entire class, including the various methods in the program. Final just means that variable shall not be changed through out the code. Private just changes visibility not accessibility. For a variable to be abstract, the method itself would need to abstract and that doesn't really influence accessibility.
Example Question #1 : Console Output
Which of the following blocks of code will output an evenly-spaced 2D array (i.e. an evenly-spaced matrix)? For example, a simple evenly-spaced matrix is:
1 2 3
4 5 6
7 8 9
int[][] matrix = {{134,135,12},{31,1,41},{-1441,14,51},{14,5,134}};
for(int i = 0; i < matrix.length; i++) {
for(int j = 0; j < matrix[i].length; j++) {
System.out.print(matrix[i][j]);
}
System.out.print(" ");
}
int[][] matrix = {{134,135,12},{31,1,41},{-14441,14,51},{14,5,134}};
for(int i = 0; i < matrix.length; i++) {
for(int j = 0; j < matrix[i].length; j++) {
if(j != 0) {
System.out.print("\t\t");
}
System.out.print(matrix[i][j]);
}
System.out.println();
}
int[][] matrix = {{134,135,12},{31,1,41},{-14441,14,51},{14,5,134}};
for(int i = 0; i < matrix.length; i++) {
for(int j = 0; j < matrix[i].length; j++) {
if(j != 0) {
System.out.print(" ");
}
System.out.print(matrix[i][j]);
}
System.out.println();
}
int[][] matrix = {{134,135,12},{31,1,41},{-14441,14,51},{14,5,134}};
for(int i = 0; i < matrix.length; i++) {
for(int j = 0; j < matrix[i].length; j++) {
if(j == 0) {
System.out.print("\t\t");
}
System.out.print(matrix[i][j]);
}
System.out.println();
}
int[][] matrix = {{134,135,12},{31,1,41},{-14441,14,51},{14,5,134}};
for(int i = 0; i < matrix.length; i++) {
for(int j = 0; j < matrix[i].length; j++) {
if(j != 0) {
System.out.print(" ");
}
System.out.println(matrix[i][j]);
}
System.out.println();
}
int[][] matrix = {{134,135,12},{31,1,41},{-14441,14,51},{14,5,134}};
for(int i = 0; i < matrix.length; i++) {
for(int j = 0; j < matrix[i].length; j++) {
if(j != 0) {
System.out.print("\t\t");
}
System.out.print(matrix[i][j]);
}
System.out.println();
}
There are several critical points to look at in the code given as possible answers above.
First, you need to make sure that the main output is not a new line. Thus, the following is incorrect:
System.out.println(matrix[i][j]);
This would create a new line for every single value!
Next, you need to make sure that a new line is output after every row of the matrix. This is the final output statement. Thus, it cannot be:
System.out.print(" ");
This would only output a character with no new line.
Finally, for everything except for the first element of each row, you will need to output sufficient padding before the given element element (so as to make the matrix to be evenly-spaced). We are not going to be too particular here about the problem of very big numbers—the values are given to us as is. Note that it is insufficient to use a mere space for this. You should use a tab character (defined as '\t'). A space will not guarantee enough spacing (no pun intended)!
The correct answer has the following logic:
if(j != 0) {
System.out.print("\t\t");
}
This means "when the column is not the first column" (i.e. j != 0), "then output tabs to space sufficiently."
Example Question #31 : Program Implementation
Consider the following code:
for(int i = 1; i <= 10; i++) {
for(int j = 0; j < (20 - i * 2) / 2; j++) {
System.out.print(" ");
}
for(int j = 0; j < i * 2; j++) {
System.out.print("*");
}
for(int j = 0; j < (20 - i * 2) / 2; j++) {
System.out.print(" ");
}
System.out.println();
}
Describe the output of the code above.
An arrow pointing upward, having a base 20 characters wide and a top that is 1 character wide.
An arrow pointing downward, having a base 20 characters wide and a top that is 2 characters wide.
An arrow pointing upward, having a base 20 characters wide and a top that is 2 characters wide.
A left-facing arrow with a large base of 20 characters in size and a point that is 1 character wide.
A right-facing arrow with large base of 20 characters in size and a point that is 2 characters wide.
An arrow pointing upward, having a base 20 characters wide and a top that is 2 characters wide.
This code is best explained by analyzing it directly. Comments will be provided in bold below:
// The loop runs from 1 to 10
for(int i = 1; i <= 10; i++) {
// First, you run through 0 to some number
// That number is computed by taking the current value of i, doubling it,
// subtracting that from 20, then dividing by 2.
// So, consider the following values for i:
// 0: 20 - 0 = 20; Divided by 2: 10
// 1: 20 - 2 = 18; Divided by 2: 9
// 2: 20 - 2 = 16; Divided by 2: 8
// etc...
// Thus, you will output single spaces that number of times (10, 9, 8...)
for(int j = 0; j < (20 - i * 2) / 2; j++) {
System.out.print(" ");
}
// Next, you will output an asterix i * 2 number of times. Thus, your
// smallest value is 2 and your largest 20.
for(int j = 0; j < i * 2; j++) {
System.out.print("*");
}
// This is just a repeat of the same loop from above
for(int j = 0; j < (20 - i * 2) / 2; j++) {
System.out.print(" ");
}
// This bumps us down to the next line
System.out.println();
}
Thus, you have output that basically has even padding on the left and right, with an increasing number of asterix characters (2, 4, 6, etc). This is an upward-facing arrow, having a point of width 2 and a base of width 20.
Example Question #181 : Computer Science
Consider the following code:
What is the output of the method call mystery("Green eggs and ham")
Green,eggs,and,ham,
Greeneggsandham,
Green eggs and ham,
,G,r,e,e,n, ,e,g,g,s, ,a,n,d, ,h,a,m,
ham,
,G,r,e,e,n, ,e,g,g,s, ,a,n,d, ,h,a,m,
The method String.split() splits a String into an array of Strings separated according to the expression within the method argument. The expression String.split("") splits the String at every character. The "for" loop concatenates the elements of the String array together, separated by a comma.
All AP Computer Science A Resources
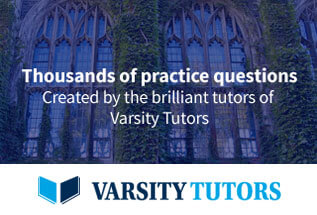